Note: I suggest reading the following tutorial as well which uses the latest Spring Security 3.1
Spring Security 3.1 - Implement UserDetailsService with Spring Data JPA
Spring Security 3.1 - Implement UserDetailsService with Spring Data JPA
What is Spring Security?
Spring Security is a powerful and highly customizable authentication and access-control framework. It is the de-facto standard for securing Spring-based applicationsWe'll build first the Spring MVC part of our application, then add Spring Security.
Spring Security is one of the most mature and widely used Spring projects. Founded in 2003 and actively maintained by SpringSource since, today it is used to secure numerous demanding environments including government agencies, military applications and central banks. It is released under an Apache 2.0 license so you can confidently use it in your projects.
Source: Spring Security
Let's declare our primary controller first.
MainController
This controller declares two mappings:
/main/common - any registered user can access this page /main/admin - only admins can access this pageEach mapping will resolve to a specific JSP page. The common JSP page is accessible by everyone, while the admin page is accessible only by admins. Right now, everyone has access to these pages because we haven't enabled Spring Security yet.
Here are the JSP pages:
commonpage.jsp
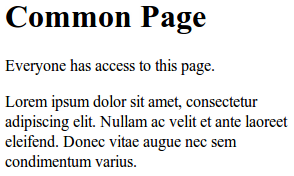
adminpage.jsp
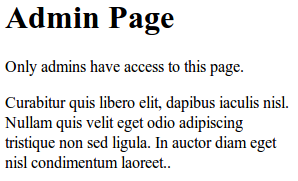
We've finished setting-up the primary controller and the associated JSP views. Now, we add the required XML configurations to enable Spring MVC and Spring Security at the same time.
We start by adding the web.xml:
web.xml
Notice the url-patterns for the DelegatingFilterProxy and DispatcherServlet. Spring Security is placed at the root-path
/*Whereas, Spring MVC is placed at a sub-path
/krams/*
We also referenced two important XML configuration files:
spring-security.xml applicationContext.xml
spring-security.xml contains configuration related to Spring Security.
spring-security.xml
The elements are self-documenting. If you're using an IDE, like Eclipse or STS, try pointing your mouse to any of these elements and you will see a short description of the element.
Notice that the bulk of the security configuration is inside the http element. Here's what we observe:
1. We declared the denied page URL in the
access-denied-page="/krams/auth/denied"
2. We provided three URLs with varying permissions. We use Spring Expression Language (SpEL) to specify the role access. For admin only access we specified hasRole('ROLE_ADMIN') and for regular users we use hasRole('ROLE_USER'). To enable SpEL, you need to set use-expressions to true
3. We declared the login URL
login-page="/krams/auth/login"
4. We declared the login failure URL
authentication-failure-url="/krams/auth/login?error=true"
5. We declared the URL where the user will be redirected if he logs out
logout-success-url="/krams/auth/login"
6. We declared the logout URL
logout-url="/krams/auth/logout"
7. We declared a default authentication-manager that references an in-memory user-service
8. We declared an in-memory user-service:
This basically declares two users with corresponding passwords and authorities. This user-service is a good way to prototype and test a Spring Security application quickly.
9. We also declared an Md5 password encoder:
When a user enters his password, it's plain string. The password we have in our database (in this case, an in-memory lists) is Md5 encoded. In order for Spring to match the passwords, it's need to encode the plain string to Md5. Once it has been encoded, then it can compare passwords.
Now we need to create a special controller that handles the login and logout requests.
LoginLogoutController
This controller declares two mappings:
/auth/login - shows the login page /auth/denied - shows the denied access pageEach mapping will resolve to a specific JSP page.
Here are the JSP pages:
loginpage.jsp
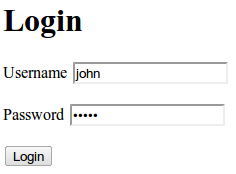
deniedpage.jsp
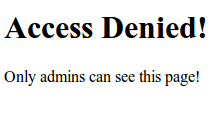
That's it. We got a working Spring MVC 3 application that's secured by Spring Security. We've managed to build a simple and quick configuration Spring Security configuration.
To access the common page, enter the following URL:
http://localhost:8080/spring-security-simple-user-service/krams/main/commonTo access the admin page, enter the following URL:
http://localhost:8080/spring-security-simple-user-service/krams/main/adminTo login, enter the following URL:
http://localhost:8080/spring-security-simple-user-service/krams/auth/loginTo logout, enter the following URL:
http://localhost:8080/spring-security-simple-user-service/krams/auth/logoutI suggest you read my other tutorial Spring Security 3 - MVC Integration Tutorial (Part 2) for comparison purposes.
The best way to learn further is to try the actual application.
Download the project
You can access the project site at Google's Project Hosting at http://code.google.com/p/spring3-security-mvc-integration-tutorial/
You can download the project as a Maven build. Look for the spring-security-simple-user-service.zip in the Download sections.
You can run the project directly using an embedded server via Maven.
For Tomcat: mvn tomcat:run
For Jetty: mvn jetty:run
Share the joy:
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |

