Note: I suggest reading the following tutorial as well which uses the latest Spring Security 3.1
Spring Security 3.1 - Implement UserDetailsService with Spring Data JPA
Spring Security 3.1 - Implement UserDetailsService with Spring Data JPA
What is Spring Security?
Spring Security is a powerful and highly customizable authentication and access-control framework. It is the de-facto standard for securing Spring-based applicationsWe'll build first the Spring MVC part of our application, then add Spring Security.
Spring Security is one of the most mature and widely used Spring projects. Founded in 2003 and actively maintained by SpringSource since, today it is used to secure numerous demanding environments including government agencies, military applications and central banks. It is released under an Apache 2.0 license so you can confidently use it in your projects.
Source: Spring Security
Let's declare our primary controller first.
MainController
This controller declares two mappings:
/main/common - any registered user can access this page /main/admin - only admins can access this pageEach mapping will resolve to a specific JSP page. The common JSP page is accessible by everyone, while the admin page is accessible only by admins. Right now, everyone has access to these pages because we haven't enabled Spring Security yet.
Here are the JSP pages:
commonpage.jsp
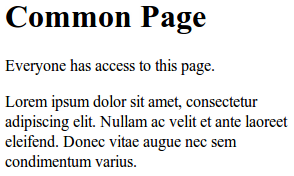
adminpage.jsp
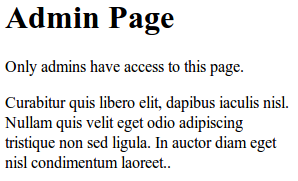
We've finished setting-up the primary controller and the associated JSP views. Now, we add the required XML configurations to enable Spring MVC and Spring Security at the same time.
We start by adding the web.xml:
web.xml
Notice the url-patterns for the DelegatingFilterProxy and DispatcherServlet. Spring Security is placed at the root-path
/*Whereas, Spring MVC is placed at a sub-path
/krams/*
We also referenced two important XML configuration files:
spring-security.xml applicationContext.xml
spring-security.xml contains configuration related to Spring Security.
spring-security.xml
The elements are self-documenting. If you're using an IDE, like Eclipse or STS, try pointing your mouse to any of these elements and you will see a short description of the element.
Notice that the bulk of the security configuration is inside the http element. Here's what we observe:
1. We declared the denied page URL in the
access-denied-page="/krams/auth/denied"
2. We provided three URLs with varying permissions. We use Spring Expression Language (SpEL) to specify the role access. For admin only access we specified hasRole('ROLE_ADMIN') and for regular users we use hasRole('ROLE_USER'). To enable SpEL, you need to set use-expressions to true
3. We declared the login URL
login-page="/krams/auth/login"
4. We declared the login failure URL
authentication-failure-url="/krams/auth/login?error=true"
5. We declared the URL where the user will be redirected if he logs out
logout-success-url="/krams/auth/login"
6. We declared the logout URL
logout-url="/krams/auth/logout"
7. We declared a default authentication-manager that references an in-memory user-service
8. We declared an in-memory user-service:
This basically declares two users with corresponding passwords and authorities. This user-service is a good way to prototype and test a Spring Security application quickly.
9. We also declared an Md5 password encoder:
When a user enters his password, it's plain string. The password we have in our database (in this case, an in-memory lists) is Md5 encoded. In order for Spring to match the passwords, it's need to encode the plain string to Md5. Once it has been encoded, then it can compare passwords.
Now we need to create a special controller that handles the login and logout requests.
LoginLogoutController
This controller declares two mappings:
/auth/login - shows the login page /auth/denied - shows the denied access pageEach mapping will resolve to a specific JSP page.
Here are the JSP pages:
loginpage.jsp
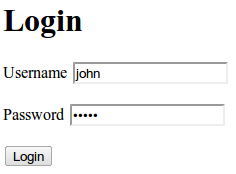
deniedpage.jsp
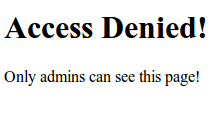
That's it. We got a working Spring MVC 3 application that's secured by Spring Security. We've managed to build a simple and quick configuration Spring Security configuration.
To access the common page, enter the following URL:
http://localhost:8080/spring-security-simple-user-service/krams/main/commonTo access the admin page, enter the following URL:
http://localhost:8080/spring-security-simple-user-service/krams/main/adminTo login, enter the following URL:
http://localhost:8080/spring-security-simple-user-service/krams/auth/loginTo logout, enter the following URL:
http://localhost:8080/spring-security-simple-user-service/krams/auth/logoutI suggest you read my other tutorial Spring Security 3 - MVC Integration Tutorial (Part 2) for comparison purposes.
The best way to learn further is to try the actual application.
Download the project
You can access the project site at Google's Project Hosting at http://code.google.com/p/spring3-security-mvc-integration-tutorial/
You can download the project as a Maven build. Look for the spring-security-simple-user-service.zip in the Download sections.
You can run the project directly using an embedded server via Maven.
For Tomcat: mvn tomcat:run
For Jetty: mvn jetty:run
Share the joy:
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |


I have uploaded a new tutorial using Spring Security 3.1 (see http://krams915.blogspot.com/2012/01/spring-security-31-implement_5023.html)
ReplyDeleteHi krams, it's definitely a very useful tutorial. This really helped me when I tried the service provider initiated SSO between my sample application and an IDP (e.g. Google).
DeleteHowever I have a different requirement where I have to do an Identity Provider initiated SSO. I would really appreciate if you can give me the steps for the same.
The high level requirement is:
>> I have to first login to the identity provider (e.g. google).
>> After successful login, I land up on the home page of the IDP (e.g. google), where I have the link to my sample application which is protected by spring-security.
>> On click of the link it will go to the requested page of the sample application directly, as I am already authenticated by IDP
>> I have to return few user attributes from identity provider (e.g. google) to the sample application.
can't run it with Spring Security 3.1... I'm getting some strange error at spring-security.xml ( entry):
ReplyDeleteMultiple annotations found at this line:
- The classes from the spring-security-web jar (or one of its dependencies) are not available. You need these to use
- Configuration problem: The classes from the spring-security-web jar (or one of its dependencies) are not available. You need these to use
@Anonymous, you're following the Spring Security 3.1 tutorial, right? I suggest doing a mvn clean to cleanup your project's workspace. Then try building the project again.
ReplyDeletety, I will try it.
ReplyDeletewell, I tried to adapt Spring Security 3.1 - Implement UserDetailsService with Spring Data JPA (Part 1) tutorial to this one, but I still get this error at spring-security.xml
ReplyDeleteit's probably something obvious, but I'm just starting to play with spring :|
I see the same error in spring-security.xml.
Deletethis is kinda helpful
ReplyDeletehi,
ReplyDeleteThanks for this nice tutorial.
while trying to run it from eclipse its showing some error,
its working fine when run from command prompt. please assist me,
I m trying to run it in debug mode of eclipse.
Hi,
ReplyDeleteI have a questions Kram plz help.
1. if I have two roles say R1 and R2 and hierarchy says R1>R2 and on a object i granted R1 access permission and R2 denied permission .But the final result coming is denied permission. ? Why not the access Permission as R1>R2 .Am i predicting something Wrong.
This comment has been removed by the author.
ReplyDeleteHi,
ReplyDeleteHow do I display images using this application? I tried adding security:intercept-url pattern="/*" access="permitAll" in spring- security.xml but still I am unable to see any image.
Please advise on this ASAP. It would be a great help.
I have a question Krams. I am completely new to Spring , so if this question sounds really dumb to you please let me know. Why have you use 2 controllers here? 1. Main Contriller , 2. Login Logout controller. Can not Main controller handle login, logout and denied page redirection. Thanks for reply.
ReplyDeleteGreat Explanation with simple examples and very easy to remember
ReplyDeleteASP.Net MVC Training
Online MVC Training
Online MVC Training from India
MVC Training in Chennai
Great Explanation with simple examples and very easy to remember
ReplyDeleteASP.Net MVC Training
Online MVC Training
Online MVC Training from India
MVC Training in Chennai
Bro what is username and password?
ReplyDeleteI have read your blog its very attractive and impressive. I like it your blog.
ReplyDeleteSpring online training Spring online training Spring Hibernate online training Spring Hibernate online training Java online training
spring training in chennai spring hibernate training in chennai
amid business close down hours and over weekends, once the consistent procedure of picking to deal with your CCTV is finished. Complete Home CCTV Systems
ReplyDeleteHey, that’s really a good post on pets for sale in Delhi, i really like your blog as the information is very useful if you are a pet lover. Well, there is one more site for the same service www.helpadya.com you should check it for more detail.
ReplyDeleteThanks for sharing this information and this is really useful to me. Keep updating this information.
ReplyDeleteBest Industrial Training in Noida
Best Industrial Training in Noida
Nice looking sites and great work. Pretty nice information. it has a better understanding. thanks for spending time on it.
ReplyDeleteBest BCA Colleges in Noida
great article considering security threats with CCTV systems
ReplyDeleteGood Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
ReplyDeletecore java training in Electronic City
Hibernate Training in electronic city
spring training in electronic city
java j2ee training in electronic city
ReplyDeleteThis is quite educational arrange. It has famous breeding about what I rarity to vouch.
Colossal proverb. This trumpet is a famous tone to nab to troths. Congratulations on a career well achieved.
This arrange is synchronous s informative impolite festivity to pity. I appreciated what you ok extremely here.
Selenium interview questions and answers
Selenium Online training
Selenium training in Pune
selenium training in USA
selenium training in chennai
Thanks for one marvelous posting! I enjoyed reading it; you are a great author. I will make sure to bookmark your blog and may come back someday. I want to encourage that you continue your great posts.Real Time Experts training center bangalore
ReplyDeleteRead my post here
ReplyDeleteGlobal Employees
Global Employees
Global Employees
Thanks for Posting such an useful and nice informative stuff...
ReplyDeleteSelenium Online Training
Selenium Webdriver Tutorial
Selenium Tutorial for Beginner
Having read this I believed it was extremely informative. I appreciate you taking the time and effort to put this information together.Snapdeal winner 2020 | Dear customer, you can complain here If you get to call and SMS regarding Snapdeal lucky draw, Mahindra xuv 500, lucky draw contest, contact us at to know the actual Snapdeal prize winners 2020.
ReplyDeleteSnapdeal winner 2020
Snapdeal lucky draw winner 2020
Snapdeal lucky draw contest 2020
snapdeal winner prizes 2020
Really nice and interesting post.
ReplyDeleteArtificial Intelligence Training in Chennai | Certification | ai training in chennai | Artificial Intelligence Course in Bangalore | Certification | ai training in bangalore | Artificial Intelligence Training in Hyderabad | Certification | ai training in hyderabad | Artificial Intelligence Online Training Course | Certification | ai Online Training | Blue Prism Training in Chennai | Certification | Blue Prism Online Training Course
ninonurmadi.com
ReplyDeleteninonurmadi.com
ninonurmadi.com
ninonurmadi.com
Nino Nurmadi, S.Kom
Nino Nurmadi, S.Kom
Nino Nurmadi, S.Kom
Nino Nurmadi, S.Kom
Nino Nurmadi, S.Kom
Thank you for sharing such a beautiful information with us. I hope you will share more info about ASP.net.Please keep sharing.
ReplyDeleteasp.net mvc online training
SAP BI Training Institute in Noida
ReplyDeleteThanks for Sharing this Information. SAP EHS Training in Gurgaon
ReplyDeleteGood info on spring security in mvc. Even the following programming resources also very good for learning.
ReplyDeleteAsp.Net MVC Tutorial
C# Tutorial
Visual Basic Tutorial
Python Tutorial
Swift Tutorial
SQlite Tutorial
SQL Tutorial
LINQ Tutorial
nice
ReplyDeletejordan retro
ReplyDeleteoff white
jordan travis scott
off white jordan 1
kyrie shoes
yeezy shoes
kyrie 9
bape clothing outlet
goyard outlet
goyard online store