What is DynamicJasper?
DynamicJasper (DJ) is an open source free library that hides the complexity of Jasper Reports, it helps developers to save time when designing simple/medium complexity reports generating the layout of the report elements automatically.
Source: http://dynamicjasper.com/
What is JasperReports?
JasperReports is the world's most popular open source reporting engine. It is entirely written in Java and it is able to use data coming from any kind of data source and produce pixel-perfect documents that can be viewed, printed or exported in a variety of document formats including HTML, PDF, Excel, OpenOffice and Word.
Source: http://jasperforge.org/projects/jasperreports
Similar with our previous tutorial. We will setup our Spring 3 MVC application first. If you need a review with Spring MVC, I suggest you read the other tutorials I've posted. Or you can also Google for related tutorials.
Here's a screenshot of the report document that we will be generating:
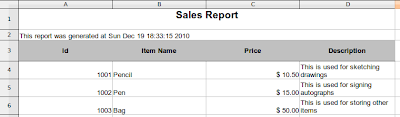
This is just basic. You can add and show more features with Jasper and DynamicJasper. For now, we'll stick with the basics.
Our first task is to setup the main controller that will handle the download request.
MainController
This controller declares two mappings:
/download - for showing the download page /download/xls - for retrieving the actual Excel report
Examine the doSalesReportXLS() controller method. It has a reference to a download service named downloadService. This service handles the actual report processing. Later, we'll discuss that in-depth.
The /download mapping will display the downloadpage view which resolves to /WEB-INF/jsp/downloadpage.jsp
downloadpage.jsp
This is a simple JSP. It has an HTML link for downloading the report. Notice the URL points to /krams/main/download/xls. The same mapping we have in the MainController.
Here's a screenshot of this page:
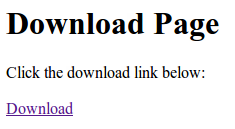
Next, we enable Spring MVC in the web.xml
web.xml
Take note of the URL pattern. When accessing any pages in our MVC application, the host name must be appended with
/krams
In the web.xml we declared a servlet-name spring. By convention, we must declare a spring-servlet.xml as well.
spring-servlet.xml
This XML config declares a view resolver. All references to a JSP name in the controllers will map to a corresponding JSP in the /WEB-INF/jsp location.
By convention, we must declare an applicationContext.xml
applicationContext.xml
This XML config declares three beans to activate the Spring 3 MVC programming model.
Let's return back to DynamicJasper.
If you remember back in the MainController we declared a reference to a DownloadService which is automatically injected by Spring.
The DownloadService is a delegate. All reporting processing is handled by this service.
DownloadService
To generate the report, we call the downloadXLS() method. This generates an Excel report. Let's examine further how the report is generated:
1. Retrieve an instance of our datasource:
SalesDAO datasource = new SalesDAO(); JRDataSource ds = datasource.getDataSource();
The datasource can come from a variety of sources like from an in-memory list, database, and alike. Later, we'll show what's inside this SalesDAO
2. Generate the Jasper layout:
ReportLayout layout = new ReportLayout(); DynamicReport dr = layout.buildReportLayout();
The layout is like the template of your report. It's basically the design of how you want the report to look like. Our Jasper layout is actually generated on the fly by DynamicJasper. That's the main purpose of DynamicJasper. It will dynamically, programmatically generate the layout. This is useful for generating tabular reports which are mostly used for professional applications. If you need to manually design or create an artistic layout, I advise you try layouting using Jasper's native JRXML format. DynamicJasper can also use a JRXML template, but that's beyond the scope of this tutorial.
3. Compile the Jasper layout into a JasperReport object:
JasperReport jr = DynamicJasperHelper.generateJasperReport(dr, new ClassicLayoutManager(), params);
The JasperReport object is the compiled version of your layout that can be understood by Jasper. Imagine it like this: the layout is for humans, while the compiled object is for the computer.
4. Create the Jasper print:
JasperPrint jp = JasperFillManager.fillReport(jr, params, ds);
This basically fills the JasperReport with contents from the datasource.
5. Export the report to your desired format. For this tutorial we will export it as an
Excel document.
ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exporter exporter = new Exporter(); exporter.export(jp, baos);
Here we pass an instance of a ByteArrayOutputStream and a JasperPrint object.
6. Set the response header and content type:
String fileName = "SalesReport.xls"; response.setHeader("Content-Disposition", "inline; filename="+ fileName); response.setContentType("application/vnd.ms-excel"); response.setContentLength(baos.size());
Make sure you set the correct contentType.
7. Write the report to the output stream:
writeReportToResponseStream(response, baos);
Now, let's examine the remaining classes.
The ReportLayout
This is a custom wrapper to DynamicJasper's FastReportBuilder. The purpose of this class is to encapsulate the layout of the report. This isn't mandatory. You can remove the contents of buildReportLayout() and place it along with the DownloadService. Take note we're encapsulating a DynamicJasper feature here, not a Jasper. So if you have specific questions about these, make sure to search the DynamicJasper forums.
The Exporter
This is a custom wrapper to Jasper's JRXlsExporter. The purpose of this class is to encapsulate the exporting of the report to different formats. This isn't mandatory. You can remove the contents of export() and place it along with the DownloadService. Take note we're encapsulating a Jasper feature here, not a DynamicJasper. So if you have specific questions about these, make sure to search the Jasper forums.
The datasource
Here we provide a custom datasource made from in-memory list of Sales items. We use the DAO name here to indicate that the data can come from a DAO or other persistence means.
SalesDAO
Our datasource returns a list of Sales. This is a simple Data Transfer Object for containing our data from the database.
Sales
Our application is now finished. We've managed to setup a simple Spring 3 MVC application. Then we added reporting using DynamicJasper. We've divided our application's concerns in various layers: controllers, services, DAO, layout, and exporter so that we can easily change implementation and custom processing. We've also leveraged Spring's MVC programming model via annotation.
To access the download page, enter the following URL:
http://localhost:8080/spring-djasper-integration/krams/main/download
If you want to download the report directly, enter the following URL:
http://localhost:8080/spring-djasper-integration/krams/main/download/xls
The best way to learn further is to try the actual application.
Download the project
You can access the project site at Google's Project Hosting at http://code.google.com/p/spring-mvc-dynamicjasper-integration-tutorial/
You can download the project as a Maven build. Look for the spring-djasper-integration.zip in the Download sections.
You can run the project directly using an embedded server via Maven.
For Tomcat: mvn tomcat:run
For Jetty: mvn jetty:run
If you want to learn more about Spring MVC and Jasper, feel free to read my other tutorials in the Tutorials section.
Share the joy:
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |


Great post! thank you for using DynamicJasper
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteHello
ReplyDeleteI have integrated Dynamic Reports with my Srpring 3.0 Project.Here i have seen some difficult to dsiplay the data in the Model
This is my POJO
public class Ent {
private long Id;
private long etId;
protected List AVA ava;
private ET et;
private long sCount;
}
I could able display all fields other than ava; This class is like bellow
public class AVA{
private String vchar;
private Long vLong;
private long eTypeId;
}
How can display my ava.vchar , ava.vLong values?
Any idea?
You'll have to make a new class where you have to explicitly write all the properties. Then you have to map the values of Ent to this new class. For example:
ReplyDeletepublic class EntDTO() {
private long Id;
private long etId;
private String vchar;
private Long vLong;
private long eTypeId;
private ET et;
private long sCount;
}
EntDTO entDTO = new EntDTO();
entDTO.setId(ent.getId())
... etc.
Ok finally i resolved in list inside list problem using Map.
ReplyDeleteNow i could able to generate reports using jasperviewer and genarate pdf,html formats. But I need display the report on my jsp (i.e in HTML format) later user could download either in pdf or what else he want. How can i displays the report on JSP page?
finally this link resolves my problem now i could able to display my report result on my jsp
ReplyDeletehttp://dynamicjasper.com/2010/10/06/how-to-export-to-html/
i need to pass the parameters in the query string dynamically to the jrxml. can u please help me out.
ReplyDeleteI added poi-3.7-20101029 jar to export xls formate report.But iam getting this error.How to resolve this?
ReplyDeletejava.lang.NoSuchMethodError: org.apache.poi.hssf.usermodel.HSSFRichTextString.applyFont(IILorg/apache/poi/hssf/usermodel/HSSFFont;)V
at net.sf.jasperreports.engine.export.JRXlsExporter.getRichTextString(JRXlsExporter.java:601)
at net.sf.jasperreports.engine.export.JRXlsExporter.setRichTextStringCellValue(JRXlsExporter.java:582)
at net.sf.jasperreports.engine.export.JRXlsExporter.createTextCell(JRXlsExporter.java:551)
at net.sf.jasperreports.engine.export.JRXlsExporter.exportText(JRXlsExporter.java:410)
at net.sf.jasperreports.engine.export.JRXlsAbstractExporter.exportPage(JRXlsAbstractExporter.java:590)
at net.sf.jasperreports.engine.export.JRXlsAbstractExporter.exportReportToStream(JRXlsAbstractExporter.java:463)
at net.sf.jasperreports.engine.export.JRXlsAbstractExporter.exportReport(JRXlsAbstractExporter.java:186)
at com.jbent.peoplecentral.model.manager.DynamicJasperManagerImpl.export(DynamicJasperManagerImpl.java:222)
at com.jbent.peoplecentral.controller.DynamicJasperReportController.export(DynamicJasperReportController.java:96)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source)
at java.lang.reflect.Method.invoke(Unknown Source)
Make sure the POI version you're using is the version compatible with Jasper and DynamicJasper. There are cases where new versions of POI aren't compatible with Jasper. In that case you have to use an older jar
ReplyDelete@Sri, check my other tutorials for Jasper/DynamicJasper integration. There's a tutorial that demonstrates how to pass an HQL query
ReplyDeleteHello
ReplyDeleteThanks for such nice tutorials.
I need to make a confirmation report in which I do not need any table
My need is to represent only one value for any field
So please tell me how can we do??
Thanks
@Gaurav, DynamicJasper is mainly designed for tabular data. If what you need is something beyond tabular, ie. a specific design, you have to do it with Jasper. Use IReport to design the report template. Try checking the following guide: http://krams915.blogspot.com/2010/12/spring-3-mvc-jasper-integration.html
ReplyDeleteHello Sir Thanks for reply
ReplyDeleteI sent you one mail on ur email id "krams915@gmail.com", please reply me
Hi krams I want to generate a pdf in a manner that it asks for save as dialogue.Can u pls help on that?
ReplyDelete@Anonymous, you can create a button "Save As Pdf" in your web application. Then on that button trigger the controller that generates the Pdf.
ReplyDeleteHello,
ReplyDeleteNice to find such tutorial , thank you very much for your efforts.
I am using jasperreport 3.7.6 with the framework seam 2 and i am doing well . Now i am trying to integrate JasperDynamic , my question is it possible to work with this latter ?
DynamicJasper should work with JasperReports regardless of your framework. If you can use Jasper, you can use DynamicJasper
DeleteThank you very much for your reply.
DeleteI appreciate your generousness and kindness profoundly.
Best wishes
I opened this project in NetBenas and run it on integrated Apache TomCat and get this error in browser:
ReplyDeleteHTTP Status 404 - /
type Status report
message /
description The requested resource (/) is not available.
Apache Tomcat/7.0.22
Any advices pls?
Very nice post. Good job.
ReplyDelete@Anonymous Use url http://localhost:8080/spring-djasper-integration/krams/main/download
hi,
ReplyDeletei have a problem that my Data object is like this
public class CandidateExcelModel {
private int id;
private String name; //varchar(50)
private String email; // varchar(100)
private Date dob; // date
private List skill =new ArrayList();
}
in this skill of list type and variable which can change time to time
need help
hi,
ReplyDeletei have a problem that my Data object is like this
public class CandidateExcelModel {
private int id;
private String name; //varchar(50)
private String email; // varchar(100)
private Date dob; // date
private List skill =new ArrayList();
}
in this skill of list type and variable which can change time to time
need help
It works great in localhost but online doesnt download the file, instead shows an 404 error
ReplyDeleteI have read your blog its very attractive and impressive. I like it your blog.
ReplyDeleteSpring online training Spring online training Spring Hibernate online training Spring Hibernate online training Java online training
spring training in chennai spring hibernate training in chennai
You should write a source in your artcles.
ReplyDeleteBest BCA Colleges in Noida
Good Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
ReplyDeletecore java training in Electronic City
Hibernate Training in electronic city
spring training in electronic city
java j2ee training in electronic city
Hi, Great.. Tutorial is just awesome..It is really helpful for a newbie like me.. I am a regular follower of your blog. Really very informative post you shared here. Kindly keep blogging.
ReplyDeleteJava training in Chennai
Java training in Bangalore
Permission to share friends, articles about health and hopefully useful for all
ReplyDeleteCara Mengatasi Telat Haid
Cara Menyembuhkan Polip Hidung
Obat Kurap
Cara Mengatasi Haid Tidak Teratur
Obat Bisul Ampuh
Amazing Post. Your writing is very inspiring. Thanks for Posting.
ReplyDeleteJava application development company
Java development company
Java outsourcing company
Hire java developer
java web development services
I have scrutinized your blog its engaging and imperative. I like it your blog.
ReplyDeletecustom application development services
Software development company
software application development company
offshore software development company
custom software development company
The article is so informative. This is more helpful for our
ReplyDeletesoftware testing training courses
selenium classes Thanks for sharing
An iOS app can be a high-productive tool for your business that can help promote your brand among greater number of consumers.
ReplyDeleteMobile app Development Company in India
This post is very impressive for me. I read your whole blog and I really enjoyed your article. Thank you...!
ReplyDeletePega Training in Chennai
Pega Course in Chennai
Excel Training in Chennai
Corporate Training in Chennai
Embedded System Course Chennai
Linux Training in Chennai
Spark Training in Chennai
Tableau Training in Chennai
Pega Training in Tambaram
Pega Training in Porur
I have to agree with everything in this post. Thanks for the useful sharing information.
ReplyDeleteRPA Training in anna nagar
RPA Training in Chennai
RPA Training in OMRR
DevOps Training in OMR
Web Designing Course in anna nagar
RPA Training in T Nagar
SEO Training in Anna Nagar
ccna Training in OMR
Best place to learn Python in Bangalore!! myTectra!!
ReplyDeletePython training in Bangalore
nice blog
ReplyDeletedata science training in bangalore
good blog
ReplyDeleteAWS Training in Bangalore
Nice article.
ReplyDeleteFor data science training in bangalore,visit:
Data science training in bangalore
For Blockchain training in Bangalore, visit:
ReplyDeleteBlockchain training in bangalore
vist here for know best devops training in bangalore
ReplyDeleteDEVOPS TRAINING IN BANGALORE
VISIT HERE => Big Data And Hadoop Training in Bangalore
ReplyDeleteThanks for Fantasctic blog and its to much informatic which i never think ..Keep writing and grwoing your self
ReplyDeleteBirth certificate in delhi
Birth certificate in ghaziabad
Birth certificate in gurgaon
Birth certificate in noida
How to get birth certificate in ghaziabad
how to get birth certificate in delhi
birth certificate agent in delhi
how to download birth certificate
birth certificate in greater noida
birth certificate agent in delhi
Birth certificate in delhi
It’s really Nice and Meaningful. It’s really cool Blog. You have really helped lots of people who visit Blog and provide them Useful Information. Thanks for Sharing.hadoop training institutes in bangalore
ReplyDeleteI am really happy to say it’s an interesting post to read. I learn new information from your article, you are doing a great job. Keep it up
ReplyDeleteUpgrade your career Learn AWS Training from industry experts get Complete hands-on Training, Interview preparation, and Job Assistance at Bangalore Training Academy Located in BTM Layout.
Thanks for sharing such a great blog Keep posting.
ReplyDeletedatabase marketing
hr contacts database
corporate directory
database providers in mumbai
b2b data companies
crm software
company database
Really very happy to say, your post is very interesting to read. I never stop myself to say something about it.You’re doing a great job. Keep it up...
ReplyDeleteSoftgen Infotech have the best Python Training in Bangalore . Any professional who is looking out to switch their career can enroll with us.
Such a great word which you use in your article and article is amazing knowledge. thank you for sharing it.
ReplyDeleteBest SAP MM Training in Bangalore - eTechno Soft Solutions is a leading SAP MM Training Institute in Bangalore offering extensive SAP MM Training by Real-time Working Professionals along with 100% placement support, Book a Free Demo!
7starhd movies
ReplyDeleteExcellent blog, keep sharing this blog. This blog contains full of usefull information..
ReplyDeleteDOT NET Training in Chennai
DOT NET Training in Bangalore
DOT NET Training Institutes in Bangalore
DOT NET Course in Bangalore
Best DOT NET Training Institutes in Bangalore
DOT NET Institute in Bangalore
DOT NET Training Institute in Marathahalli
PHP Training in Bangalore
Spoken English Classes in Bangalore
Data Science Courses in Bangalore
It was a great blog with so much information of the beautiful places to visit...Sarkari Result has currently published jobs like India Post GDS Online Form, Bank of Maharashtra Recruitment,, AIIMS recruitment, MPPKVVCL Recruitment, Indian Navy Recruitment, Vizag Steel and many more. So, never miss a chance to check out Sarkari Result on daily basis for getting all the information about RRB Recruitment 2020 .
ReplyDeletei have been following this website blog for the past month. i really found this website was helped me a lot and every thing which was shared here was so informative and useful. again once i appreciate their effort they are making and keep going on.
ReplyDeleteDigital Marketing Consultant in Chennai
Freelance Digital Marketing Consultant
Wow!! Really a nice Article about Selenium. Thank you so much for your efforts. Definitely, it will be helpful for others. I would like to follow your blog. Share more like this. Thanks Again.
ReplyDeleteJava training in chennai | Java training in annanagar | Java training in omr | Java training in porur | Java training in tambaram | Java training in velachery
Effective blog with a lot of information. I just Shared you the link below for Courses .They really provide good level of training and Placement,I just Had Spring Classes in this institute,Just Check This Link You can get it more information about the Spring course.
ReplyDeleteJava training in chennai | Java training in annanagar | Java training in omr | Java training in porur | Java training in tambaram | Java training in velachery
This is truly unique and excellent information. I sense you think a lot like me, or vice versa. Thank you for sharing this great article.
ReplyDeleteSAP training in Kolkata
SAP training Kolkata
Best SAP training in Kolkata
SAP course in Kolkata
Expressing thanks to you for partaking such immensities of information within few momentous sentences in this content. I am really looking forward to read some more motivating articles.
ReplyDeleteSoftware Testing Training in Bangalore
Software Testing Training
Software Testing Online Training
<
Software Testing Training in Hyderabad
Software Testing Courses in Chennai
Software Testing Training in Coimbatore
This is such a great resource that you are providing and you give it away for free! good presentation skills | Virtual team building | Piano Lessons Singapore
ReplyDeleteGreat information!!! Thanks for your wonderful informative blog.
ReplyDeleteVillage Talkies a top-quality professional corporate video production company in Bangalore and also best explainer video company in Bangalore & animation video makers in Bangalore, Chennai, India & Maryland, Baltimore, USA provides Corporate & Brand films, Promotional, Marketing videos & Training videos, Product demo videos, Employee videos, Product video explainers, eLearning videos, 2d Animation, 3d Animation, Motion Graphics, Whiteboard Explainer videos Client Testimonial Videos, Video Presentation and more for all start-ups, industries, and corporate companies. From scripting to corporate video production services, explainer & 3d, 2d animation video production , our solutions are customized to your budget, timeline, and to meet the company goals and objectives.
As a best video production company in Bangalore, we produce quality and creative videos to our clients.
This comment has been removed by the author.
ReplyDeleteyeezy outlet
ReplyDeletegolden goose outlet
bape
goyard
birkin bag
yeezy shoes
golden goose
yeezy
jordan shoes
off white
wonderful blog. It's very interesting to read...
ReplyDeleteC Programming course at Edukators in Coimbatore