Note: I suggest reading the following updated tutorial instead:
Spring MVC 3.1 and JasperReports: Using iReport and AJAX
Spring MVC 3.1 and JasperReports: Using iReport and AJAX
What is Jasper Reports?
JasperReports is the world's most popular open source reporting engine. It is entirely written in Java and it is able to use data coming from any kind of data source and produce pixel-perfect documents that can be viewed, printed or exported in a variety of document formats including HTML, PDF, Excel, OpenOffice and Word.What is iReport?
Source: http://jasperforge.org/projects/jasperreports
iReport is the free, open source report designer for JasperReports. Create very sophisticated layouts containing charts, images, subreports, crosstabs and much more. Access your data through JDBC, TableModels, JavaBeans, XML, Hibernate, CSV, and custom sources. Then publish your reports as PDF, RTF, XML, XLS, CSV, HTML, XHTML, text, DOCX, or OpenOffice.Similar with our previous tutorial. We will setup our Spring 3 MVC application first. If you need a review with Spring MVC, I suggest you read the other tutorials I've posted. Or you can also Google for related tutorials.
Source: http://jasperforge.org/index.php?q=project/ireport
Here's a screenshot of the report that we will be generating:
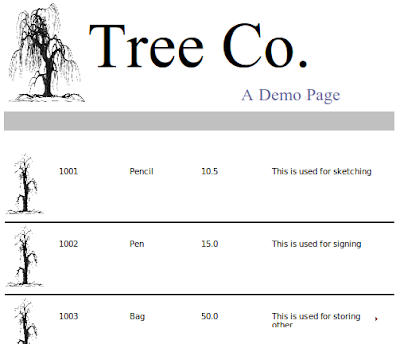
This is just basic. You can add more features such as charts,custom calculations, and other report related functions with Jasper. If you need to design the layout, you can use iReport to do the job.
Our first task is to setup the main controller that will handle the download request.
This controller declares three mappings:
/main/download - for showing the download page /main/download/xls - for retrieving the actual Excel report /main/download/pdf - for retrieving the actual PDF reportTo add support for HTML and CSV formats, just copy any of the methods. Rename the value of the @RequestMapping and follow the pattern. I will leave that part as an exercise for my reader.
In each request handler, we return the datasource:
We then placed the datasource in the a Map:
Then we added it to the ModelAndView.
For XLS, we did the following:
modelAndView = new ModelAndView("xlsReport", parameterMap);
And for PDF, we did the following:
modelAndView = new ModelAndView("pdfReport", parameterMap);
The only thing that changed are the view names: xlsReport and pdfReport. These view names are declared on a separate XML file under /WEB-INF/jasper-views.xml
jasper-views.xml
We've declared four beans in this config. Each bean uses a Spring built-in view for Jasper that corresponds to a specific format. For example, JasperReportsXlsView is used for rendering Excel reports.
Each report has a reportDataKey that references the datasource from the controller. It also has a reference to the Jasper template tree-template.jrxml
Let's complete our Spring MVC setup.
To enable Spring MVC we need add it in the web.xml
web.xml
Take note of the URL pattern. When accessing any pages in our MVC application, the host name must be appended with
/krams
In the web.xml we declared a servlet-name spring. By convention, we must declare a spring-servlet.xml as well.
spring-servlet.xml
Notice we declared two view resolvers here: an InternalResourceViewResolver and an XmlViewResolver. If you've been following my other tutorials, you'll notice that we always declare an InternalResourceViewResolver only.
We must declare an extra view resolver this time to help Spring to choose the correct view to render our reports. If we just use InternalResourceViewResolver, all view references will point to /WEB-INF/jsp/ and will be appended with a .jsp extension. We don't want that because that location is for JSPs. Our report views are internal within Spring's package and we indicate that inside the /WEB-INF/jasper-views.xml file.
We're done with the spring-servlet.xml file.
By convention, we must declare an applicationContext.xml as well.
applicationContext.xml
This XML config declares three beans to activate the Spring 3 MVC programming model.
Now we move to the crucial point of our tutorial. Remember earlier we had a reference to a template file named tree-template.jrxml
tree-template.jrxml (a partial view)
If you notice our report template is just plain XML! Nothing magical here. Actually we used a special tool to generate this XML template. It's called iReport. To get started with this tool, visit the Getting Started guide from JasperForge.
For this tutorial, we need to do the following:
1. Download and install iReport.
2. Run iReport
3. Go to File. Then click on New
4. Select the Tree template . Any template will do, even a blank one.
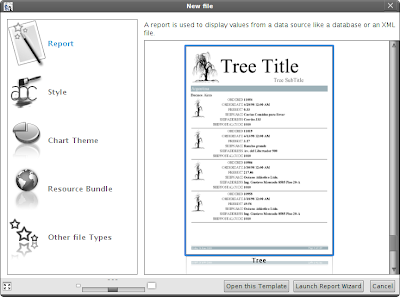
You should see a ready-made template:
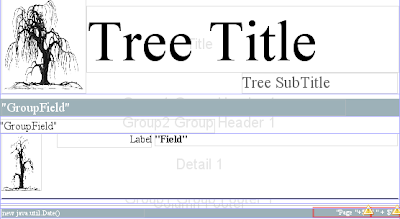
5. We need to add four new fields in this layout. On the upper-left side, click on Fields.
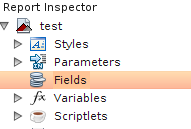
6. Right-click and add four fields:
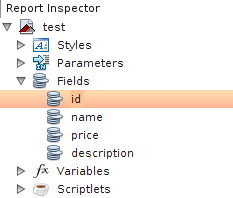
7. Make sure to set the correct types:
id: Long name: String price: Double description: String
On the upper-right side, there's a panel where you can modify the properties of the fields we declared.
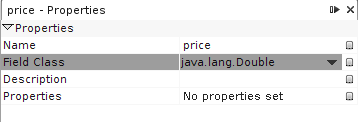
8. Now we need to drag each of those fields to the main layout. See below:

9. Then modify the title and subtitle. This isn't really mandatory. Just make sure you placed those four fields.
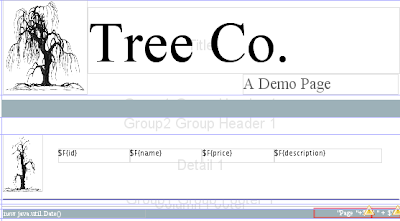
10. Click on the main layout again. On the right-side panel, you should see a list of properties for the main report layout. You need to change the default Language from Groovy to Java. Failure to do so will result to multiple compilation errors. Unless you know Groovy and familiar with its dependencies, it's best you stick with Java for now.
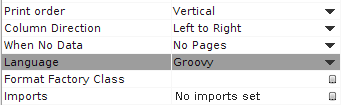
11. Save the document.
12. Now you need to locate that document. Copy and place it in the classpath of your web application. If you look carefully on the directory where the original document is saved, there are two images that had been saved as well. Make sure to copy them as well! Failure to copy them to your classpath will cause an Exception in the application.
Here's how our final directory should look like:
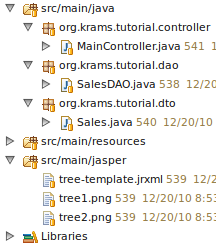
Remember we declared four fields in this layout. Where exactly do we get our data to fill-in these fields? It's assigned by the controller.
See that line:
JRDataSource datasource = dataprovider.getDataSource();That's where we get our data.
The datasource
Here we provide a custom datasource made from in-memory list of Sales items. We use the DAO name here to indicate that the data can come from a DAO or other persistence means.
SalesDAO
Our datasource returns a list of Sales. This is a simple Data Transfer Object for containing our data from the database.
Sales
Our application is now finished. We've managed to setup a simple Spring 3 MVC application. Then we added reporting using Jasper. We use Spring's built-in support to render the Jasper views. We've used iReport to design the report document. We've also leveraged Spring's MVC programming model via annotation.
To access the download page, enter the following URL:
http://localhost:8080/spring-djasper-integration/krams/main/download
Here's a final look to our download page:
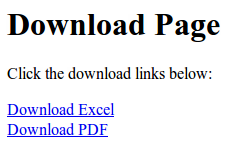
downloadpage.jsp
If you want to download the report directly, enter the following URL for XLS format:
http://localhost:8080/spring-jasper-integration/krams/main/download/xls
For PDF format, enter the following URL:
http://localhost:8080/spring-jasper-integration/krams/main/download/pdf
For HTML and CSV, I left this exercise for my readers.
The best way to learn further is to try the actual application.
Download the project
You can access the project site at Google's Project Hosting at http://code.google.com/p/spring-mvc-jasper-integration-tutorial/
You can download the project as a Maven build. Look for the spring-jasper-integration.zip in the Download sections.
You can run the project directly using an embedded server via Maven.
For Tomcat: mvn tomcat:run
For Jetty: mvn jetty:run
If you want to learn more about Spring MVC and Jasper, feel free to read my other tutorials in the Tutorials section.
Share the joy:
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |


Nice tutorial,
ReplyDeleteIf you already have a dataSource configured you can inject it as a "jdbcDataSource" property
it was help full i could run the report but its opening in the same window.........
ReplyDeletei want to open it in a new window........
any idea
target attribute for <a> element is what you are looking for ;-)
Deletehttp://www.w3schools.com/tags/att_a_target.asp
thanks, helped me a lot this tutorial, but I fail to see the images when I change the report to HTML. Has anyone done it?
ReplyDeletegot same problem
DeleteGot the same problem and struggling alot.If you get the solution can you post it here.
DeleteSame problem with HTML - but great tutorial
Deletethanks, very concise and helpful
ReplyDeletethanks dude, it was really helpful... this is best when it compared with other hectic tutorials.... keep it up.. - B.A.L.Ajith Kumara
ReplyDeleteThank you very much for publishing these tutorials. They are very helpful.
ReplyDeleteIt doesn't work :( page download/pdf is not found.
ReplyDeleteIt renders as a jsp file so it's not found
ReplyDeletetutorial very good and hopefully can help me in building a web application thanks
ReplyDeletewhenever i try to download the excel file.. i encounter this problem..
ReplyDeletejava.lang.ClassNotFoundException: org.springframework.ui.jasperreports.JasperReportsUtils
what can be the cause?
org.springframework
spring-support
2.0.6
i m not able to run the project, m using jetty server please explain me in brikef any one...
ReplyDeleteHello
ReplyDeleteThanks for such nice tutorials.
I need to make a confirmation report in which I do not need any table
My need is to represent only one value for any field
So please tell me how can we do??
Thanks
Great article. Thanks a lot!
ReplyDeleteI imported this as a Maven Project. I tried to run this on server and I get the message ""the resource spring-jasper-integration not found".
ReplyDeleteCan u help me with this?
My pdf report is not getting the proper name.I want to set proper name to my report.Any one have an idea.
ReplyDelete'i keep getting a pdfreport.jsp is not available' error
ReplyDeletehi all, i am newbie for jasper, when i download this example i got an error like
ReplyDeleteSEVERE: Error configuring application listener of class org.springframework.web.context.ContextLoaderListener
java.lang.ClassNotFoundException: org.springframework.web.context.ContextLoaderListener
can anybody help on this
thanks to all
ramesh-hyd
Nice example.. Thanks.
ReplyDeleteThe most important thing here I guess is the language in iReport.
By default, it is Groovy, needs to be changed to Java.
I spent almost 3-4 hours untill I saw this blog.
How I can define a single view (JasperReportsPdfView) for multiple jasper templates (*. jrxml)?
ReplyDeleteHave you looked at the following guide: http://krams915.blogspot.com/2012/01/spring-mvc-31-and-jasperreports-using_5116.html ?
DeleteHello,
ReplyDeleteHow add other parameters to report.
thank you for this posting!
ReplyDeletenow i have to make bills for client.
u alrady know about my english skill is sosososososo bad.
actually my english is suck!!
but i can understand everything about Jasper.
i would like to say ur posting is sosososososo easy!!
have a good day!!
Hi,
ReplyDeleteI am struggling to integrate different characters in my xls report. I used code 2000 and chinese character from DB are coming fine in PDF. But when exporting in xls format it shows blankk.. Can you help?
-Thanks
I am compiling this project, and after this project is not found
ReplyDeleteAnybody help me ?
Thank you
This comment has been removed by the author.
ReplyDeletehi
ReplyDeletewhenever i try to download the pdf file.. i encounter this problem..
nested exception is java.lang.NoSuchMethodError: com.lowagie.text.pdf.PdfWriter.setRgbTransparencyBlending(Z)V
with iText-2.1.7 and jasperreports-5.1.0
what can be the cause?
Thank you
how to give filename dynamically to jasper reports...
ReplyDeletePlease can someone tell me how to pass parameters instead of a datasource ? ...
ReplyDeleteWhat can be the response type if I try to call the get service by the use of resttemplate as it returns ModelView?
ReplyDeleteEx:
reportRestTemplate.get("/demoReport",???????);
~~~~~~What should I submit as response type?
i got this error
ReplyDeleteCould not resolve view with name 'pdfReport' in servlet with name 'spring'
how can i solve this??
Hello Krams sir,
ReplyDeleteThank you. I usually follows your tutorials. I need your help in creating Spring MVC MongoDB Jasper integration example. Could you please create it?
You should write a source in your artcles.
ReplyDeleteBest BCA Colleges in Noida
I am really impressed with your efforts and really pleased to visit this post.
ReplyDeleteData Science with Python training in chenni
Data Science training in chennai
Data science training in velachery
Data science training in tambaram
Data Science training in OMR
Data Science training in anna nagar
Data Science training in chennai
Data science training in Bangalore
Whoa! I’m enjoying the template/theme of this website. It’s simple, yet effective. A lot of times it’s very hard to get that “perfect balance” between superb usability and visual appeal. I must say you’ve done a very good job with this.
ReplyDeleteAWS Online Training | Online AWS Certification Course - Gangboard
Best Selenium Training in Chennai | Selenium Training Institute in Chennai | Besant Technologies
Selenium Training in Bangalore | Best Selenium Training in Bangalore
AWS Training in Bangalore | Amazon Web Services Training in Bangalore
Amazon Web Services Training in Pune | Best AWS Training in Pune
you have done a meritorious work by posting this content.
ReplyDeleteBest selenium training in chennai
Best Selenium Training Institute in Chennai
iOS Training in Chennai
French Classes in Chennai
Big Data Training in Chennai
Big Data Certification
Big Data Training
Thanks for sharing this useful information. Keep doing regularly.
ReplyDeleteIELTS Classes in Chennai
Best IELTS Courses in Chennai
IELTS in Chennai
Best IELTS Class in Chennai
IELTS Training Institute in Chennai
IELTS Coaching Classes in Chennai
Best IELTS Institute in Chennai
Your good knowledge and kindness in playing with all the pieces were very useful. I don’t know what I would have done if I had not encountered such a step like this.
ReplyDeletedevops online training
aws online training
data science with python online training
data science online training
rpa online training
Well Said, you have furnished the right information that will be useful to anyone at all time. Thanks for sharing your Ideas.
ReplyDeleteMicrosoft Azure online training
Selenium online training
Java online training
uipath online training
Python online training
ReplyDeleteYour very own commitment to getting the message throughout came to be rather powerful and have consistently enabled employees just like me to arrive at their desired goals.
Java Training in Chennai | Best Java Training in Chennai
C C++ Training in Chennai | Best C C++ Training in Chennai
Attend The Python training in bangalore From ExcelR. Practical Python training in bangalore Sessions With Assured Placement Support From Experienced Faculty. ExcelR Offers The Python training in bangalore.
ReplyDeletepython training in bangalore
I appreciate your hard work. It was a beautiful blog with more informative article. Hydraulic elevators | lifts for home
ReplyDelete
ReplyDeleteI am really impressed with the way of writing of this blog.I really appreciate your hard work. It was a beautiful blog with more informative article. Keep it up!!
artificial intelligence course
This comment has been removed by the author.
ReplyDeleteI learned World's Trending Technology from certified experts for free of cost. I Got a job in decent Top MNC Company with handsome 14 LPA salary, I have learned the World's Trending Technology from Data Science Training in btm experts who know advanced concepts which can help to solve any type of Real-time issues in the field of Python. Really worth trying High PR Social Bookmarking Submissions Sites List 2019
ReplyDeleteFor AI training in Bangalore, Visit:
ReplyDeleteArtificial Intelligence training in Bangalore
For AI training in Bangalore, Visit:- Artificial Intelligence training in Bangalore
ReplyDeleteVery interesting blog Thank you for sharing such a nice and interesting blog and really very helpful article.python training in bangalore
ReplyDeleteIts really helpful for the users of this site. I am also searching about these type of sites now a days. So your site really helps me for searching the new and great stuff.vmware training in bangalore
ReplyDeleteReally it was an awesome article,very interesting to read.You have provided an nice article,Thanks for sharing.informatica training in bangalore
ReplyDeleteEnjoyed reading the article above, really explains everything in detail, the article is very interesting and effective. Thank you and good luck…
ReplyDeleteUpgrade your career Learn SharePoint Developer Training in Bangalore from industry experts get Complete hands-on Training, Interview preparation, and Job Assistance at Softgen Infotech.
Great post. Your blog is quite helpful to me and i am sure to others too. Automatic aluminum Trackless
ReplyDeleteGreat Post
ReplyDeletewonderful thanks for sharing an amazing idea. keep it...
ReplyDeleteSoftgen Infotech have the best Python Training in Bangalore . Any professional who is looking out to switch their career can enroll with us.
I am happy for sharing on this blog its awesome blog I really impressed. thanks for sharing.
ReplyDeleteBest SAP MM Training in Bangalore - eTechno Soft Solutions is a leading SAP MM Training Institute in Bangalore offering extensive SAP MM Training by Real-time Working Professionals along with 100% placement support, Book a Free Demo!
Nice post...
ReplyDeleteAustralia hosting
Bermuda web hosting
Botswana hosting
mexico web hosting
moldova web hosting
albania web hosting
andorra hosting
armenia web hosting
australia web hosting
good post
ReplyDeletedenmark web hosting
inplant training in chennai
I have read your excellent post. Thanks for sharing
ReplyDeleteaws Training in Bangalore
python Training in Bangalore
hadoop Training in Bangalore
angular js Training in Bangalore
bigdata analytics Training in Bangalore
python Training in Bangalore
aws Training in Bangalore
nice....................
ReplyDeletevietnam web hosting
google cloud server hosting
canada telus cloud hosting
algeeria hosting
angola hostig
shared hosting
bangladesh hosting
botswana hosting
central african republi hosting
shared hosting
nice post...very useful for learning students...
ReplyDeletepython training in chennai
internships in hyderabad for cse 2nd year students
online inplant training
internships for aeronautical engineering students
kaashiv infotech internship review
report of summer internship in c++
cse internships in hyderabad
python internship
internship for civil engineering students in chennai
robotics course in chennai
It is very good and useful for students and developer.Learned a lot of new things from your post Good creation,thanks for give a good information.
ReplyDeletesalesforce developer training in bangalore
salesforce developer courses in bangalore
salesforce developer classes in bangalore
salesforce developer training institute in bangalore
salesforce developer course syllabus
best salesforce developer training
salesforce developer training centers
I am really happy with your blog because your article is very unique and powerful for new reader.
ReplyDeleteaws Training in Bangalore
python Training in Bangalore
hadoop Training in Bangalore
angular js Training in Bangalore
bigdata analytics Training in Bangalore
python Training in Bangalore
aws Training in Bangalore
I am really happy with your blog because your article is very unique and powerful for new reader.
ReplyDeleteaws Training in Bangalore
python Training in Bangalore
hadoop Training in Bangalore
angular js Training in Bangalore
bigdata analytics Training in Bangalore
python Training in Bangalore
aws Training in Bangalore
This post is really nice and informative. The explanation given is really comprehensive and informative. artificial intelligence course syllabus by 10+ years experienced faculty.
ReplyDeleteReally awesome blog!!!
ReplyDeleteData Science Course
Data Science Course in Marathahalli
Pretty article! I found some useful information in your blog, it was awesome to read, learn azure thanks for sharing this great content to my vision, keep sharing.
ReplyDeleteYour blog is quite helpful to me and i am sure to others too. I appreciate your post,. Home lifts Dubai
ReplyDeleteGreat Article… I love to read your articles because your writing style is too good, they are becomes a more and more interesting from the starting lines until the end.
ReplyDeleteVacuum lifts
its is very very helpful for all of us and I never get bored while reading your article because, Elite Elevators are the best home lifts in India. | Home lifts Malaysia
ReplyDeletenice imformation....
ReplyDeletecoronavirus update
inplant training in chennai
inplant training
inplant training in chennai for cse
inplant training in chennai for ece
inplant training in chennai for eee
inplant training in chennai for mechanical
internship in chennai
online internship
ReplyDeleteThis is most informative and also this post most user friendly and super navigation to all posts. Thank you so much for giving this information to me.Artificial Intelligence training in Chennai.
Java training in chennai | Java training in annanagar | Java training in omr | Java training in porur | Java training in tambaram | Java training in velachery
This blog is the general information for the feature. You got a good work for these blog.We have a developing our creative content of this mind.Thank you for this blog. This for very interesting and useful.
ReplyDeleteArtificial Intelligence Training in Chennai
Ai Training in Chennai
Artificial Intelligence training in Bangalore
Ai Training in Bangalore
Artificial Intelligence Training in Hyderabad | Certification | ai training in hyderabad
Artificial Intelligence Online Training
Ai Online Training
Blue Prism Training in Chennai
ReplyDeleteamazing post written ... It shows your effort and dedication. Thanks for share such a nice post.Java training in Chennai
Java Online training in Chennai
Java Course in Chennai
Best JAVA Training Institutes in Chennai
Java training in Bangalore
Java training in Hyderabad
Java Training in Coimbatore
Java Training
Java Online Training
Excellent Blog! I would Thanks for sharing this wonderful content.its very useful to us.I gained many unknown information, the way you have clearly explained is really fantastic.keep posting such useful information.
ReplyDeleteFull Stack Training in Chennai | Certification | Online Training Course
Full Stack Training in Bangalore | Certification | Online Training Course
Full Stack Training in Hyderabad | Certification | Online Training Course
Full Stack Developer Training in Chennai | Mean Stack Developer Training in Chennai
Full Stack Training
Full Stack Online Training
Hire Asp .Net Developerhas the propelled aspect of Micro's.Net structure and it is viewed as the best application system for building dynamic sites and online applications. Subsequently the cutting edge asp.net development companies advancement organizations that have dynamic website specialists and online application engineers profoundly depend on this. The accompanying focuses made ASP .Net a default decision for everybody. asp .net web development company (articulated speck net) is a system that gives a programming rules that can be utilized to build up a wide scope of uses – from web to portable to Windows-based applications. The dot net development companies in chennaisystem can work with a few programming dialects, for example, C#, VB.NET, C++ and F#. At Grand Circus, we use C#.
ReplyDeleteNice information, valuable and excellent design, as share good stuff with good ideas and concepts, lots of great information and inspiration, both of which I need, thanks to offer such a helpful information here.
ReplyDeleteIELTS Coaching in chennai
German Classes in Chennai
GRE Coaching Classes in Chennai
TOEFL Coaching in Chennai
spoken english classes in chennai | Communication training
I feel really happy to have seen your webpage.I am feeling grateful to read this.you gave a nice information for us.please updating more stuff content...keep up!!
ReplyDeleteAndroid Training in Chennai
Android Online Training in Chennai
Android Training in Bangalore
Android Training in Hyderabad
Android Training in Coimbatore
Android Training
Android Online Training
I feel really happy to have seen your webpage and look forward to so many more entertaining times reading here. Thanks once more for all the details.
ReplyDeleteacte chennai
acte complaints
acte reviews
acte trainer complaints
acte trainer reviews
acte velachery reviews complaints
acte tambaram reviews complaints
acte anna nagar reviews complaints
acte porur reviews complaints
acte omr reviews complaints
Great post! I am see the great contents and step by step read really nice information.I am gather this concepts and more information. It's helpful for me my friend. Also great blog here with all of the valuable information you have.
ReplyDeleteAWS Course in Chennai
AWS Course in Bangalore
AWS Course in Hyderabad
AWS Course in Coimbatore
AWS Course
AWS Certification Course
AWS Certification Training
AWS Online Training
AWS Training
Thanks for sharing Good Information
ReplyDeletePython Interview Question And Answers
Web Api Interview Questions
OSPF Interview Questions And Answers
Thank you.. This is very helpful
ReplyDeleteData science Training in bangalore | Data science Live Online Training
Aws Training In Bangalore | Aws Live Online Training
Hadoop Training In Bangalore | Hadoop Live Online Training
Devops Training In Bangalore | Devops Live Online Training
IOT Training in Bangalore | IOT Live Online Training
Hello! This is my first visit to your blog! We are a team of volunteers and starting a new initiative in a community in the same niche.
ReplyDelete| Certification | Cyber Security Online Training Course | Ethical Hacking Training Course in Chennai | Certification | Ethical Hacking Online Training Course | CCNA Training Course in Chennai | Certification | CCNA Online Training Course | RPA Robotic Process Automation Training Course in Chennai | Certification | RPA Training Course Chennai | SEO Training in Chennai | Certification | SEO Online Training Course
buy juicy fruit online
ReplyDeletebuy gelato strain online
Buy dark star strain online
buy hawaiian skunk strain online
buy bc big bud strain leafly
buy auto flowering seeds online
buy brass knuckles vape recall 2018
buy alaskan thunder fuck online
buy cannabis seeds bank online
what is contrave
ReplyDeletesilicon wives
sky pharmacy
atx 101 uk
macrolane buttock injections london
hydrogel buttock injections
buying vyvanse online legit
buy dermal fillers online usa
mesotherapy injections near me
xeomin reviews
Great blog.thanks for sharing such a useful information
ReplyDeleteSalesforce CRM Training in Chennai
cloud payroll software
ReplyDeleteintegrated payroll systems
leave management system
ONLEI Technologies
ReplyDeletePython Training
Machine Learning
Data Science
Digital Marketing
Industrial Training In Noida
Winter Training In Noida
IT Training
Payroll Software
ReplyDeletepayroll software singapore
Hi, Thanks for sharing nice articles...
ReplyDeleteImage Data Entry
This post is so interactive and informative.keep update more information...
ReplyDeleteTally Course in Velachery
Tally course in Chennai
ReplyDeleteorganic chemistry notes
Mindblowing blog very useful thanks
ReplyDeletedot net training in T Nagar
Dot net training in Chennai
organic chemistry notes
ReplyDeletegamsat organic chemistry
cbse organic chemistry
iit organic chemistry
Great post. keep sharing such a worthy information.
ReplyDeleteMobile Application Testing Online Training
ReplyDeleteIt is different from the data insight aspect. Algorithms are used to develop data, whereas the executives make better decisions about the product using data insight.
data science course in lucknow
Thank you For you Article.UJR Technologies provides Digital marketing services in KPHBwith best price.that promotes your business strength and increase sales revenue.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteVery Informative blog.
ReplyDeleteHindi calligraphy fonts download