Note: I suggest reading the following tutorial as well which uses the latest Spring Security 3.1
Spring Security 3.1 - Implement UserDetailsService with Spring Data JPA
Spring Security 3.1 - Implement UserDetailsService with Spring Data JPA
What is Spring Security?
Spring Security provides comprehensive security services for J2EE-based enterprise software applications. There is a particular emphasis on supporting projects built using The Spring Framework, which is the leading J2EE solution for enterprise software development. If you're not using Spring for developing enterprise applications, we warmly encourage you to take a closer look at it. Some familiarity with Spring - and in particular dependency injection principles - will help you get up to speed with Spring Security more easily.Let's start by creating a special controller that handles the login and logout requests.
Source: http://static.springsource.org/spring-security/site/docs/3.1.x/reference/introduction.html#what-is-acegi-security
LoginLogoutController
package org.krams.tutorial.controller; import org.apache.log4j.Logger; import org.springframework.stereotype.Controller; import org.springframework.ui.ModelMap; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; /** * Handles and retrieves the login or denied page depending on the URI template */ @Controller @RequestMapping("/auth") public class LoginLogoutController { protected static Logger logger = Logger.getLogger("controller"); /** * Handles and retrieves the login JSP page * * @return the name of the JSP page */ @RequestMapping(value = "/login", method = RequestMethod.GET) public String getLoginPage(@RequestParam(value="error", required=false) boolean error, ModelMap model) { logger.debug("Received request to show login page"); // Add an error message to the model if login is unsuccessful // The 'error' parameter is set to true based on the when the authentication has failed. // We declared this under the authentication-failure-url attribute inside the spring-security.xml /* See below: <form-login login-page="/krams/auth/login" authentication-failure-url="/krams/auth/login?error=true" default-target-url="/krams/main/common"/>*/ if (error == true) { // Assign an error message model.put("error", "You have entered an invalid username or password!"); } else { model.put("error", ""); } // This will resolve to /WEB-INF/jsp/loginpage.jsp return "loginpage"; } /** * Handles and retrieves the denied JSP page. This is shown whenever a regular user * tries to access an admin only page. * * @return the name of the JSP page */ @RequestMapping(value = "/denied", method = RequestMethod.GET) public String getDeniedPage() { logger.debug("Received request to show denied page"); // This will resolve to /WEB-INF/jsp/deniedpage.jsp return "deniedpage"; } }This controller declares two mappings:
/auth/login - shows the login page /auth/denied - shows the denied access pageEach mapping will resolve to a specific JSP page.
Here are the JSP pages:
loginpage.jsp
This is a simple HTML form and input elements. If you need a review, please visit the HTML Forms and Input tutorials from w3schools. We have two text input elements:
j_username j_passwordThese are Spring's placeholder for the username and password respectively.
When the form is submitted, it will be sent to the following action URL:
j_spring_security_checkTake note of the EL expression
${error}This is used for displaying invalid credentials during login. The value is derived from the returned model of the controller. See our controller declaration below.
Next we create the access denied page. This page will be displayed if the user is trying to access an unauthorized page. For example, a regular user tries to access an admin only page will get an access denied page.
deniedpage.jsp
Here's how the JSP pages should look like:
loginpage.jsp
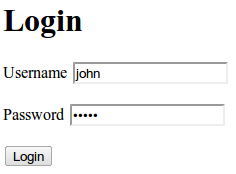
deniedpage.jsp
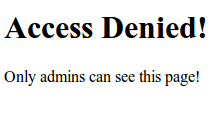
We've finished the login/logout controller and the associated JSP views. We will now enable Spring Security in our application.
To enable it, we need to the following steps:
1. Add a DelegatingFilterProxy in the web.xml
2. Declare a custom XML config named spring-security.xml
In the web.xml we declare an instance of a DelegatingFilterProxy. This basically filters requests based on the declared url-pattern.
web.xml
Notice the url-patterns for the DelegatingFilterProxy and DispatcherServlet. The Spring Security is placed at the root-path
/*Whereas, Spring MVC is placed at a sub-path
/krams/*We also referenced two important XML configuration files:
spring-security.xml applicationContext.xmlspring-security.xml contains configuration related to Spring Security.
spring-security.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:security="http://www.springframework.org/schema/security" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/security http://www.springframework.org/schema/security/spring-security-3.0.xsd"> <!-- This is where we configure Spring-Security --> <security:http auto-config="true" use-expressions="true" access-denied-page="/krams/auth/denied" > <security:intercept-url pattern="/krams/auth/login" access="permitAll"/> <security:intercept-url pattern="/krams/main/admin" access="hasRole('ROLE_ADMIN')"/> <security:intercept-url pattern="/krams/main/common" access="hasRole('ROLE_USER')"/> <security:form-login login-page="/krams/auth/login" authentication-failure-url="/krams/auth/login?error=true" default-target-url="/krams/main/common"/> <security:logout invalidate-session="true" logout-success-url="/krams/auth/login" logout-url="/krams/auth/logout"/> </security:http> <!-- Declare an authentication-manager to use a custom userDetailsService --> <security:authentication-manager> <security:authentication-provider user-service-ref="customUserDetailsService"> <security:password-encoder ref="passwordEncoder"/> </security:authentication-provider> </security:authentication-manager> <!-- Use a Md5 encoder since the user's passwords are stored as Md5 in the database --> <bean class="org.springframework.security.authentication.encoding.Md5PasswordEncoder" id="passwordEncoder"/> <!-- A custom service where Spring will retrieve users and their corresponding access levels --> <bean id="customUserDetailsService" class="org.krams.tutorial.service.CustomUserDetailsService"/> </beans>The elements are self-documenting. If you're using an IDE, like Eclipse or STS, try pointing your mouse to any of these elements and you will see a short description of the element.
Notice that the bulk of the security configuration is inside the http element. Here's what we observe:
1. We declared the denied page URL in the
access-denied-page="/krams/auth/denied"
2. We provided three URLs with varying permissions. We use Spring Expression Language (SpEL) to specify the role access. For admin only access we specified hasRole('ROLE_ADMIN') and for regular users we use hasRole('ROLE_USER'). To enable SpEL, you need to set use-expressions to true
3. We declared the login URL
login-page="/krams/auth/login"
4. We declared the login failure URL
authentication-failure-url="/krams/auth/login?error=true"
5. We declared the URL where the user will be redirected if he logs out
logout-success-url="/krams/auth/login"
6. We declared the logout URL
logout-url="/krams/auth/logout"
7. We declared an authentication-manager that references a custom user-service
8. We declared a custom user-service
9. We also declared an Md5 password encoder:
When a user enters his password, it's plain string. The password we have in our database (in this case, an in-memory lists) is Md5 encoded. In order for Spring to match the passwords, it need's to encode the plain string to Md5. Once it has been encoded, then it can compare passwords.
Let's focus for a moment to the custom user-service.
To allow Spring to use our custom database schema, we need to provide a custom user-service. This service must implement Spring's UserDetailsService interface.
CustomUserDetailsService
package org.krams.tutorial.service; import java.util.ArrayList; import java.util.Collection; import java.util.List; import org.apache.log4j.Logger; import org.krams.tutorial.dao.UserDAO; import org.krams.tutorial.domain.DbUser; import org.springframework.dao.DataAccessException; import org.springframework.security.core.GrantedAuthority; import org.springframework.security.core.authority.GrantedAuthorityImpl; import org.springframework.security.core.userdetails.User; import org.springframework.security.core.userdetails.UserDetails; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework.security.core.userdetails.UsernameNotFoundException; import org.springframework.transaction.annotation.Transactional; /** * A custom service for retrieving users from a custom datasource, such as a database. * <p> * This custom service must implement Spring's {@link UserDetailsService} */ @Transactional(readOnly = true) public class CustomUserDetailsService implements UserDetailsService { protected static Logger logger = Logger.getLogger("service"); private UserDAO userDAO = new UserDAO(); /** * Retrieves a user record containing the user's credentials and access. */ public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException, DataAccessException { // Declare a null Spring User UserDetails user = null; try { // Search database for a user that matches the specified username // You can provide a custom DAO to access your persistence layer // Or use JDBC to access your database // DbUser is our custom domain user. This is not the same as Spring's User DbUser dbUser = userDAO.searchDatabase(username); // Populate the Spring User object with details from the dbUser // Here we just pass the username, password, and access level // getAuthorities() will translate the access level to the correct role type user = new User( dbUser.getUsername(), dbUser.getPassword().toLowerCase(), true, true, true, true, getAuthorities(dbUser.getAccess()) ); } catch (Exception e) { logger.error("Error in retrieving user"); throw new UsernameNotFoundException("Error in retrieving user"); } // Return user to Spring for processing. // Take note we're not the one evaluating whether this user is authenticated or valid // We just merely retrieve a user that matches the specified username return user; } /** * Retrieves the correct ROLE type depending on the access level, where access level is an Integer. * Basically, this interprets the access value whether it's for a regular user or admin. * * @param access an integer value representing the access of the user * @return collection of granted authorities */ public Collection<grantedauthority> getAuthorities(Integer access) { // Create a list of grants for this user List<grantedauthority> authList = new ArrayList<grantedauthority>(2); // All users are granted with ROLE_USER access // Therefore this user gets a ROLE_USER by default logger.debug("Grant ROLE_USER to this user"); authList.add(new GrantedAuthorityImpl("ROLE_USER")); // Check if this user has admin access // We interpret Integer(1) as an admin user if ( access.compareTo(1) == 0) { // User has admin access logger.debug("Grant ROLE_ADMIN to this user"); authList.add(new GrantedAuthorityImpl("ROLE_ADMIN")); } // Return list of granted authorities return authList; } }This custom service implements the loadUserByUsername() method and provides a getAuthorities() method for retrieving authorities.
The purpose of loadUserByUsername() method is to return an instance of a fully populated Spring User object. It's up to you how you retrieve the data. In this tutorial, we retrieve the user by searching a custom DAO.
The purpose of getAuthorities() method is to translate our custom access level to a Spring Security GrantedAuthority representation. Remember our custom database stores access levels as integers. Spring Security interprets authorities based on GrantedAuthority() representations.
We just perform a simple if-else condition and return the corresponding authority:
Our custom database is accessible through a dummy DAO implementation:
UserDAO
package org.krams.tutorial.dao; import java.util.ArrayList; import java.util.List; import org.apache.log4j.Logger; import org.krams.tutorial.domain.DbUser; /** * A custom DAO for accessing data from the database. * */ public class UserDAO { protected static Logger logger = Logger.getLogger("dao"); /** * Simulates retrieval of data from a database. */ public DbUser searchDatabase(String username) { // Retrieve all users from the database ListThis dummy DAO doesn't really connect to a database. It just provides an in-memory list of users. If you just need to setup a simple in-memory user-service, please read my other tutorial Spring Security 3 - MVC: Using a Simple User-Service Tutorialusers = internalDatabase(); // Search user based on the parameters for(DbUser dbUser:users) { if ( dbUser.getUsername().equals(username) == true ) { logger.debug("User found"); // return matching user return dbUser; } } logger.error("User does not exist!"); throw new RuntimeException("User does not exist!"); } /** * Our fake database. Here we populate an ArrayList with a dummy list of users. */ private List internalDatabase() { // Dummy database // Create a dummy array list List users = new ArrayList (); DbUser user = null; // Create a new dummy user user = new DbUser(); user.setUsername("john"); // Actual password: admin user.setPassword("21232f297a57a5a743894a0e4a801fc3"); // Admin user user.setAccess(1); // Add to array list users.add(user); // Create a new dummy user user = new DbUser(); user.setUsername("jane"); // Actual password: user user.setPassword("ee11cbb19052e40b07aac0ca060c23ee"); // Regular user user.setAccess(2); // Add to array list users.add(user); return users; } }
Furthermore, we use a custom domain object DbUser to represent the user's credentials derived from the database. If you look at the CustomUserDetailsService again, we mapped DbUser to Spring's User object.
What's Spring User?
Models core user information retrieved by a UserDetailsService.Here's DbUser:
Implemented with value object semantics (immutable after construction, like a String). Developers may use this class directly, subclass it, or write their own UserDetails implementation from scratch.
Source: Spring Security 3 API for User
DbUser
package org.krams.tutorial.domain; /** * User domain */ public class DbUser { /** * The username */ private String username; /** * The password as an MD5 value */ private String password; /** * Access level of the user. * 1 = Admin user * 2 = Regular user */ private Integer access; public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public Integer getAccess() { return access; } public void setAccess(Integer access) { this.access = access; } }To access the common page, enter the following URL:
http://localhost:8080/spring-security-integrationkrams/main/commonTo access the admin page, enter the following URL:
http://localhost:8080/spring-security-integrationkrams/main/adminTo login, enter the following URL:
http://localhost:8080/spring-security-integrationkrams/auth/loginTo logout, enter the following URL:
http://localhost:8080/spring-security-integrationkrams/auth/logout
If you like to disable Spring Security in this application, just remove the following configuration in the web.xml:
That's it. We got a working Spring MVC 3 application that's secured by Spring Security. We've also managed to authenticate our users using a custom data provider.
The best way to learn further is to try the actual application.
Download the project
You can access the project site at Google's Project Hosting at http://code.google.com/p/spring3-security-mvc-integration-tutorial/
You can download the project as a Maven build. Look for the spring-security-integration.zip in the Download sections.
You can run the project directly using an embedded server via Maven.
For Tomcat: mvn tomcat:run
For Jetty: mvn jetty:run
If you want to learn more about Spring MVC and Spring Security, feel free to read my other tutorials in the Tutorials section.
References:
Spring Security 3.1.0.M2 API
Spring Security Reference Documentation
Share the joy:
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |


Thank you so much for this.
ReplyDeleteIndeed, great work here. I have used spring security and found it awesome. you may also like this LDAP authentication example in Spring Security. if you use declarative method give in article its just few lines of xml that's it.
DeleteHi Guys,
DeleteThis is one awesome blog. Much thanks again. Fantastic.
We have a requirement like, we send the DocuSign request from and we check whether the request is successful or not from both Desktop and Mobile versions. In Desktop version, we are printing the page level err message after getting the failure response from DocuSign but the same thing we want to achieve it in mobile side as well. I know we can't print the page level messages in mobile but we can give some alert to the user on the failure side. Can someone please help me on how to get the call back response in this mobile context?
But great job man, do keep posted with the new updates.
Many Thanks,
Clinton Kevin
Great tutorial. This has very useful information. Finally I have founded the right solution for my question. Thank you.
DeleteBest Android Training Institute in Chennai
Android Training in Chennai
Welcome :)
ReplyDeleteThank you very much! I've been looking for something explaining the proper use of spring-security, and your tutorial took care of everything I wanted to know.
ReplyDeleteWell done!
Perfect, thanks
ReplyDeleteHi Krams, what is the different between
ReplyDeletespring-security.xml and spring-security-simplified.xml
Thank you!
ReplyDeleteThank you!
Thank you!
Krams for president :)
Is it possible to inject private UserDAO userDAO = new UserDAO(); via spring bean and @Resource . Because i tried that its not working
ReplyDelete@Anonymous, the following will not work because it's not managed by Spring:
ReplyDeleteprivate UserDAO userDAO = new UserDAO()
You have to declare UserDAO as @Component or via XML config. Then you can use @Autowired or @Resource to inject the DAO
ขอบคุณค้าบ..
ReplyDeleteGood job!
ReplyDeleteVery nice to read - with lots of explanations.
I like how you manage to pace on with the details without skipping too much, like I have seen on many other blogs.
thanks alote
ReplyDeleteHi krams, please write a book that summarize all of yours tutorials, you are great man !
ReplyDeleteHats off to you Krams, this is an outstanding tutorial, very concise and easy to follow - you should take aphilippe's comment about a book seriously :)
ReplyDeleteVery Good Job...
ReplyDeleteeasy to understand.
I am new to Spring security.
I have one question here! We can authenticate with access permissions using a single helper class also. So, What is the main goal of using Spring security here ?
Please let me know.
Thanks for the comments guys. Writing a book is big task :) That's why I preferred blogging for the meantime instead. But who knows it might happen. A compendium of common solutions (not just for Spring Security) sounds a good idea
ReplyDelete@Anonymous, what helper class are you referring at? The power of Spring Security is you can augment different authentication mechanisms just by declaring appropriate configuration classes. For this tutorial, the main goal is to show how you integrate Spring Security and Spring MVC. The final goal will vary from user to user.
ReplyDeleteHi krams,
ReplyDeleteVery very good job. I am going to work on new project which uses Camel Integration framework for routing/mediation with Spring and Maven. If you could please provide me initial setup and some sample programs it will be really helpful for me.
Thanks,
Raj
Awesome man!
ReplyDeleteHi,
ReplyDeleteI got working this example. I am facing one problem. I logged in with user 'jane' still I can access "http://localhost:8080/spring-security-integrationkrams/main/admin" page. Please help.
Hm... is it just me or a bug? I downloaded, unzipped the zip file, then run mvn tomcat:run. I logged in with john/admin at http://localhost:8080/spring-security-integration/krams/auth/login, then logged out at http://localhost:8080/spring-security-integration/krams/auth/logout. The browser was not re-directed to the login page, but showed this error:
ReplyDeletejava.lang.NullPointerException
java.util.Hashtable.get(Hashtable.java:334)
org.apache.tomcat.util.http.Parameters.getParameterValues(Parameters.java:195)
org.apache.tomcat.util.http.Parameters.getParameter(Parameters.java:240)
org.apache.catalina.connector.Request.getParameter(Request.java:1065)
org.apache.catalina.connector.RequestFacade.getParameter(RequestFacade.java:355)
javax.servlet.ServletRequestWrapper.getParameter(ServletRequestWrapper.java:158)
org.springframework.security.web.authentication.AbstractAuthenticationTargetUrlRequestHandler.determineTargetUrl(AbstractAuthenticationTargetUrlRequestHandler.java:86)
org.springframework.security.web.authentication.AbstractAuthenticationTargetUrlRequestHandler.handle(AbstractAuthenticationTargetUrlRequestHandler.java:67)
org.springframework.security.web.authentication.logout.SimpleUrlLogoutSuccessHandler.onLogoutSuccess(SimpleUrlLogoutSuccessHandler.java:28)
org.springframework.security.web.authentication.logout.LogoutFilter.doFilter(LogoutFilter.java:100)
org.springframework.security.web.FilterChainProxy$VirtualFilterChain.doFilter(FilterChainProxy.java:381)
org.springframework.security.web.context.SecurityContextPersistenceFilter.doFilter(SecurityContextPersistenceFilter.java:79)
org.springframework.security.web.FilterChainProxy$VirtualFilterChain.doFilter(FilterChainProxy.java:381)
org.springframework.security.web.FilterChainProxy.doFilter(FilterChainProxy.java:168)
org.springframework.web.filter.DelegatingFilterProxy.invokeDelegate(DelegatingFilterProxy.java:237)
org.springframework.web.filter.DelegatingFilterProxy.doFilter(DelegatingFilterProxy.java:167)
Does it happen to anybody?
You'll see the above exceptions if you use spring security 3.0.6.RELEASE, but the 3.0.5.RELEASE works fine. Interestingly, the code generated by spring roo 1.1.5 works fine with security 3.0.6.
ReplyDeleteHi Krams,
ReplyDeleteI am impressed by your comprehension on such a difficult subject. More importantly in the way you have interpreted it in such a clear and simple way and generously given it back to the community.
Maybe you can setup a donation section so the community can help support and encourage great people like you.
All the best and thank you for making my life easier.
Are you sure that it works?
ReplyDeleteI have alreayd a User object which have many fields , what you suggests to do? I must use Spring Secuitry User Object?
ReplyDeleteMapping it like you did?
How can I know after a user loged-in, the user details? I want to do action with the user-session-object?
THanks , and great site
I continue my question from above , does combine this example with Spring Security - MVC: Querying the SessionRegistry exmaple will get my user-object-session answer?
ReplyDelete@Anonymous, if you have a custom User object with a different set of fields, I suggest you create a Custom Authentication manager. You can check the following as a starting point: http://krams915.blogspot.com/2010/12/spring-security-mvc-integration-using_26.html. After a user logs-in, you can access his details via the SecurityContextHolder. For example to get the name: SecurityContextHolder.getContext().getAuthentication().getName()
ReplyDelete@Anonymous, you should be able to get the session information from the SessionRegistry as noted in the Spring docs: "You can list a user's sessions by calling the getAllSessions(Object principal, boolean includeExpiredSessions) method, which returns a list of SessionInformation objects."
ReplyDeletekrams , thanks for your reply, I think I might stay with customUserDetailsService without Custom Authentication and to get user from db just by mapping it back to UserDb from a Controller :
ReplyDeleteUser user = (User)SecurityContextHolder.getContext().getAuthentication().getPrincipal();
String name = user.getUsername(); //get logged in username
DbUser u = userDAO.getUser(name);
(source from mkyong)
What your opinion on this?
Does Custom Authentication way has a benefit than this?
Thanks
Instead of mapping back to DbUser , which will cause many calls to the database , is there a way to save Dbuser for example into the session? attach my object? without using Custom Authentication Manager? ( using this guide with customUserDetailsService )
ReplyDeletethanks
Hi Krams,
ReplyDeleteI get a null pointer exception for loadUserByUsername when I try to load the user information from an actual database.
Based on this tutorial, I tried to store the user information in a mysql database using JPA/Hibernate. In the service layer I added the following:
public Member getByUsername( String username ) {
return (Member) entityManager.createQuery("FROM Member m where m.username = ?username").setParameter("username", username).getSingleResult();
}
I created a search method in the UserController which uses getByUsername with not problems. Yay!
In CustomUserDetailsService the loadUserByUsername calls the same getByUsername and this time I get a NullPointerException. Boo!
When I look into the entityManager query in the logs I can see it is now returning null.
Is this something you have come across before?
I don't get why the same query works with a search but not when authenticating a user on login using loadUserByUsername.
Okay - null pointer sorted. I hadn't initialized the entity manager in the CustomUserDetails.
ReplyDeleteI have found that after logging out, I can hit the back button and still access the site normally. Is anyone else able to do this or have I screwed something up?
@Stryder, sorry for the delayed reply. I'm somewhat busy working on some projects. I'm checking right now one of my projects and yes even if you logged out, you can still access the page. But in my application, you only see the layout but you don't see the contents. That's because I added method level security (also Spring).
ReplyDeleteWhy is the page still appearing after pressing the back button of your browser? I think it's because it has been cached. Check also this thread:
http://forum.springsource.org/showthread.php?107711-Spring-Security-Logout-Back-Button-Page-History
Luke Taylor advises the reader to check the FAQ @http://static.springsource.org/spring-security/site/faq/faq.html#faq-cached-secure-page
@Stryder, from that FAQ, we have the following:
ReplyDelete1.4. Why can I still see a secured page even after I've logged out of my application?
"The most common reason for this is that your browser has cached the page and you are seeing a copy which is being retrieved from the browsers cache. Verify this by checking whether the browser is actually sending the request (check your server access logs, the debug log or use a suitable browser debugging plugin such as “Tamper Data” for Firefox). This has nothing to do with Spring Security and you should configure your application or server to set the appropriate Cache-Control response headers. Note that SSL requests are never cached."
@krams, I found the issue with logging out and still having access to some pages using the browser back button. And guess what, the problem was me. I screwed up again! Your tutorial worked perfectly, I had a typo in my intercept-url pattern. Because there were no errors I didn't think I had anything wrong.
ReplyDeleteI also had a read about the caching issue which is very interesting. Tampa Data is a useful tool too.
I can only imagine how busy you would be and I don't expect anything if I post something here. Sometimes I get to sort out my issues while I am posting. I guess this one made it to a post. Either way I appreciate your thoughts.
Thank you for the great tutorial.
very good tutorial, nice work!
ReplyDeleteWhen building with maven, maven seems to not see the security archives. In eclipse it works fine.
ReplyDeleteHere is pom:
3.0.5.RELEASE
org.springframework.security
spring-security-core
${org.springframework.version}
jar
compile
org.springframework.security
spring-security-config
${org.springframework.version}
jar
compile
org.springframework.security
spring-security-web
${org.springframework.version}
jar
compile
The libs ar collected and lies in repository.
Hi
ReplyDeleteI want to attach this to an real DB, like mysql, instead of using the internal database feature. How would you do this? And, do you have an example of it?
Thanks for an very good tutorial site..!!
Br
Frank
Great work, like all tutorials i found here..Manny thx!
ReplyDeleteThanks a lot, you're the best. I put your web into my bookmarks :)
ReplyDeleteI have uploaded a new tutorial using Spring Security 3.1 (see http://krams915.blogspot.com/2012/01/spring-security-31-implement_5023.html)
ReplyDeletegreat job krams! ;)
ReplyDeletesuper work :)
ReplyDeleteHi Krams,
ReplyDeleteI am getting 404 error when i submit the login page (/auth/login) the below is the uri it try to hit,
http://localhost:8080/Spring3_security/j_spring_security_check
can you please help if any more configuration or anything is needed?
Hi krams,
ReplyDeleteThanks for the article..
I would like to know one thing, my application currently using org.springframework.security.userdetails.jdbc.JdbcDaoImpl for authentication.
Directly injecting the usersByUsernameQuery and authoritiesByUsernameQuery in security.xml files.
Initially we were using passwords as plain text, later changed to LdapSha encoding method.
I would like to know what all things to be changed for handling this encoded password.
- Hari
Load full user data before password check. Great idea.
ReplyDeleteI prefer this way
http://krams915.blogspot.com/2010/12/spring-security-mvc-integration-using_26.html
You should also check my tutorial for Spring Security 3.1
DeleteThank you, a thousand times thank you!
ReplyDeleteHi Krams
ReplyDeleteEach time I click on any link, it takes me back to login page. Any idea on how to do configuration for that?
Basically i want to access multiple pages after login and each time i login and click on any link it send me back to login page. can u help?
Thanks
There are a lot of tutorial out there, this is definitely one of the best.
ReplyDeleteThanks!
Great tutorial. Really helped me get started.
ReplyDeleteDoes it work right though?
If I bring the web app up (in Eclipse Jave EE) and login as Jane, then try to go to the admin page I am denied access.
I then "logout" by going to the logout page which brings me to the login page. If I login as John, and go to the admin page I am able to access the page.
Finally, I logout John and login as Jane and I am now able to access the admin page (as Jane).
I assume I should be denied access. Thoughts? Suggestions?
If Jane is not an admin, she should be denied. Did you check the logs for any specific errors?
DeleteHi ,
ReplyDeleteGood work..
I have an issue while using spring security 3.
I am getting following exception
Caused by: java.lang.NoSuchMethodException: $Proxy41.isEraseCredentialsAfterAuthentication()
at java.lang.Class.getMethod(Class.java:1622)
at org.springframework.util.MethodInvoker.prepare(MethodInvoker.java:178)
at org.springframework.beans.factory.config.MethodInvokingFactoryBean.afterPropertiesSet(MethodInvokingFactoryBean.java:149)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.invokeInitMethods(AbstractAutowireCapableBeanFactory.java:1514)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.initializeBean(AbstractAutowireCapableBeanFactory.java:1452)
Can you please help here.
This comment has been removed by the author.
ReplyDeleteHi Mark,
ReplyDeleteYou are doing amazing job.
I tried many of your examples but this example, I couldn't implement properly due to some reason which I am not able to understand.
No matter what I type in login form(user or admin)..it just route me to common page which also default-target-url.
After playing with it I came to know that intercepts URL are not called so as a result it is getting routed to default url.
Could you please explain what might be wrong?
Thanks in advance
vijaya, have you checked the more updated version of this guide: http://krams915.blogspot.com/2012/01/spring-security-31-implement_5023.html ?
DeleteAlso, I suggest enabling debug logging to see the error.
@Mark,I did not check that yet.I will check that now.
ReplyDeleteI have also enabled debug mode but no help :-( .
Was trying different ways but couldn't succeed.
But thank you so much for your reply.
Awesome! This is your first post i have read. I appreciate the minute details you have covered..In one go everything worked perfectly fine in my pet project.I just followed steps shown above.Such a time saver.Thank You.
ReplyDeleteI want to use my own one-way encryption Algorithm instead of springs Md5PasswordEncoder. Can you please make me understand what should be method signature so that spring can pass user password to it for encoding before comparing with value got from DB and how can i configure it in Spring Security XML
ReplyDeleteRahul, you can pass a different password encoder under the authentication-manager element. See Part III of this tutorial http://krams915.blogspot.com/2012/01/spring-security-31-implement_5023.html I suggest you take a look at that guide. It's newer and I think cleaner.
Deleteya but line 54 clearly is doing dbUser.getPassword().toLowerCase(),
ReplyDeleteI think it should be to username. not password
Hi, nice tutorial, I need to add spring security to a small project of mine, after I added:
ReplyDeletespringSecurityFilterChain
org.springframework.web.filter.DelegatingFilterProxy
springSecurityFilterChain
/*
to web.xml, I get this error:
feb 05, 2014 3:34:48 PM org.apache.catalina.core.StandardContext filterStart
Grave: Exception starting filter springSecurityFilterChain
org.springframework.beans.factory.NoSuchBeanDefinitionException: No bean named 'springSecurityFilterChain' is defined
at org.springframework.beans.factory.support.DefaultListableBeanFactory.getBeanDefinition(DefaultListableBeanFactory.java:529)
at org.springframework.beans.factory.support.AbstractBeanFactory.getMergedLocalBeanDefinition(AbstractBeanFactory.java:1095)
at org.springframework.beans.factory.support.AbstractBeanFactory.doGetBean(AbstractBeanFactory.java:277)
at org.springframework.beans.factory.support.AbstractBeanFactory.getBean(AbstractBeanFactory.java:197)
at org.springframework.context.support.AbstractApplicationContext.getBean(AbstractApplicationContext.java:1097)
at org.springframework.web.filter.DelegatingFilterProxy.initDelegate(DelegatingFilterProxy.java:326)
at org.springframework.web.filter.DelegatingFilterProxy.initFilterBean(DelegatingFilterProxy.java:236)
at org.springframework.web.filter.GenericFilterBean.init(GenericFilterBean.java:194)
at org.apache.catalina.core.ApplicationFilterConfig.initFilter(ApplicationFilterConfig.java:281)
at org.apache.catalina.core.ApplicationFilterConfig.getFilter(ApplicationFilterConfig.java:262)
at org.apache.catalina.core.ApplicationFilterConfig.(ApplicationFilterConfig.java:107)
at org.apache.catalina.core.StandardContext.filterStart(StandardContext.java:4775)
at org.apache.catalina.core.StandardContext.startInternal(StandardContext.java:5452)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
at org.apache.catalina.core.ContainerBase.addChildInternal(ContainerBase.java:901)
at org.apache.catalina.core.ContainerBase.addChild(ContainerBase.java:877)
at org.apache.catalina.core.StandardHost.addChild(StandardHost.java:633)
at org.apache.catalina.startup.HostConfig.deployDescriptor(HostConfig.java:656)
at org.apache.catalina.startup.HostConfig$DeployDescriptor.run(HostConfig.java:1635)
at java.util.concurrent.Executors$RunnableAdapter.call(Executors.java:471)
at java.util.concurrent.FutureTask.run(FutureTask.java:262)
at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1145)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:615)
at java.lang.Thread.run(Thread.java:744)
can you help me?
thanks
mvn jetty:run does not work for me unless I include a bunch of configuration for the plugin in your pom.xml. Am I the only person experiencing this?
ReplyDeleteI’m impressed with the surpassing and instructive blogs that you just provide in such very short timing.
ReplyDeleteadt security reviews
Hi, thank for your article, but I can't run any project of you.
ReplyDeleteIt seems Tomcat dont read any of spring xml config file.
Hi this is vignesh i am having 3 years of experience as a android
ReplyDeletedeveloper and i am certified. i have knowledge on OOPS concepts in
android but dont know indepth. After learning hadoop will be enough to
get a good career in IT with good package? and i crossed hadoop training in chennai website where someone
please help me to identity the syllabus covers everything or not??
Thanks,
vignesh
ReplyDeleteHi, this is Ganesh I am having 3 years of experience as a Dot Net developer and I am certified. I have Knowledge on OOPS Concepts in .NET indepth. After learning Salesforce will be enough to get a good career in IT with good Package? and i crossed Salesforce Training in Chennai website where someone please help me to identify the syllabus covers everything or not??
Thanks,Ganesh
ReplyDeleteHi this is dwarakesh i am having 3 years of experience as a php developer and i am certified. i have knowledge on OOPS concepts in php but dont know
indepth. After learning java will be enough to get a good career in IT with good package? and i crossed
java training in Chennai website where someone please help me to identity the syllabus covers everything or not??
thanks, dwarakesh
Hi this is Ganesh i am having 3 years of experience as a java developer and i am certified. i have knowledge on OOPS concepts in java but dont know indepth. After learning php will be enough to get a good career in IT with good package? and i crossed php training in chennai website where someone please help me to identity the syllabus covers everything or not??
ReplyDeletethanks,Ganesh.
Hi this is Ganesh i am having 3 years of experience as a java developer and i am certified. i have knowledge on OOPS concepts in java but dont know indepth. After learning php will be enough to get a good career in IT with good package? and i crossed php training in chennai website where someone please help me to identity the syllabus covers everything or not??
ReplyDeletethanks,Ganesh.
Thanks for sharing this informative blog. FITA provides Salesforce Training in Chennai with years of experienced professionals and fully hands-on classes. Salesforce is a cloud based CRM software. Today's most of the IT industry use this software for customer relationship management. To know more details about salesforce reach FITA Academy. Rated as No.1 Salesforce Training Institutes in Chennai.
ReplyDeleteYour information is really useful for me. If anyone wants to get SEO Training in Chennai visit FITA Academy located at Chennai. Rated as No.1 SEO Training institutes in Chennai.
ReplyDeleteYour posts is really helpful for me.Thanks for your wonderful post.It is really very helpful for us and I have gathered some important information from this blog.If anyone wants to get Dot Net Training in Chennai reach FITA, rated as No.1 Dot Net Training Institutes in Chennai.
ReplyDeleteHi, I wish to be a regular contributor of your blog. I have read your blog. Your information is really useful for beginner. Recently I have completed Software Testing Course in Chennai at a reputed institutes.Its really useful for me to make a bright carrer. If anyone want to get Manual Testing Training in Chennai reach FITA.
ReplyDeleteThanks for sharing this informative blog. If anyone wants to get Unix Training Chennai, Please visit Fita Academy located at Chennai, Velachery.
ReplyDeleteYour blog is really awesome. Thanks for sharing this blog. If anyone want to get PHP Training in Chennai, please visit Fita academy located at Chennai, Velachery.
ReplyDeleteIt was really a wonderful article and I was really impressed by reading this blog. We are giving all software Course Online Training. The HTML5 Training Institutes in Chennai is one of the reputed Training institute in Chennai. They give professional and real time training for all students.
ReplyDeleteI am looking to create a Chrome Extension with Spring and OAuth 2. Can any one help me out? If the user has a Google account the login should be achieved through it
ReplyDeleteSalesforce Course in Chennai
ReplyDeleteI have read your blog and i got a very useful and knowledgeable information from your blog.You have done a great job . If anyone want to get Salesforce Training in Chennai, Please visit FITA academy located at Chennai Velachery.
Salesforce Developer Training in Chennai
Salesforce Administrator Training in Chennai
Hadoop Training Chennai
ReplyDeleteHi, I am Christina lives in Chennai. I am technology freak. I did Hadoop Training in Chennai at FITA. This is useful for me to make a bright career in IT field.
Hadoop Course in Chennai
Your information about Selenium scripts is really interesting. Also I want to know the latest new techniques which are implemented in selenium. Can you please update it in your website? Selenium Training in Chennai | Best Selenium training institute in Chennai | Selenium Training
ReplyDelete.
ReplyDeleteThanks for your informative article on ios mobile application development. Your article helped me to explore the future of mobile apps developers. Having sound knowledge on mobile application development will help you to float in mobile application development. iOS Training | iOS Training Institutes in Chennai
ReplyDeleteI get a lot of great information from this blog. Thank you for your sharing this informative blog. Just now I have completed hadoop certification course at a leading academy. If you are interested to learn Hadoop Training in Chennai visit FITA IT training and placement academy which offer Big Data Training in Chennai.
ReplyDeleteSalesforce Training
ReplyDeleteThe information you posted here is useful to make my career better keep updates..I did Salesforce Training in Chennai at FITA academy. Its really useful for me to make bright future in IT industry.
Salesforce CRM Training in Chennai | Salesforce Admin Training in Chennai | Salesforce.com Training in Chennai | Sales Cloud Consultant Training in Chennai
The information you posted here is useful to make my career better keep updates..If anyone want to become an oracle certified professional reach FITA Oracle Training Institutes in Chennai, which offers Best Oracle Training Chennai with years of experienced professionals.
ReplyDeleteThis is extremely helpful info!! Very good work. Everything is very interesting to learn and easy to understood. Thank you for giving information.
ReplyDeletekids games online
friv 2
unblocked games
juegos de un show mas
The information you posted here is useful to make my career better keep updates..
ReplyDeleteOracle Training in chennai
Your blog is really usefull for me.. Thanks for sharing..
ReplyDeleteOracle Training in Chennai
I have read your blog and i got a very useful and knowledgeable information from your blog.its really a very nice article.You have done a great job .
ReplyDeleteHadoop Training in Chennai
Thanks for your informative article on SAS Training. Your article helped me to explore the future...
ReplyDeleteSAS Training in Chennai
Your blog is really awesome. Thanks for sharing this blog.
ReplyDeleteGreen Technologies In Chennai
Green Technologies In Chennai
I am reading your post from the beginning, it was so interesting to read & I feel thanks to you for posting such a good blog, keep updates regularly.
ReplyDeleteRegards,
sas training in Chennai|sas course in Chennai|sas training institute in Chennai
This comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDelete
ReplyDeleteOur HP Quick Test Professional course includes basic to advanced level and our QTP course is designed to get the placement in good MNC companies in chennai as quickly as once you complete the QTP certification training course.
if learned in this site.what are the tools using in sql server environment and in warehousing have the solution thank .. msbi training In Chennai
ReplyDelete
ReplyDeletehai If you are interested in asp.net training, our real time working.
asp.net Training in Chennai.
Asp-Net-training-in-chennai.html
Hai if our training additional way as (IT) trained as individual,you will be able to understand other applications more quickly and continue to build your skll set
ReplyDeletewhich will assist you in getting hi-tech industry jobs as possible in future courese of action..
visit this blog webMethods-training in chennai
great article!!!!!This is very importent information for us.I like all content and information.I have read it.You know more about this please visit again.
ReplyDeleteQTP Training in Chennai
very nice blogs!!! i have to learning for lot of information for this sites...Sharing for wonderful information.Thanks for sharing this valuable information to our vision. You have posted a trust worthy blog keep sharing.
ReplyDeleteInformatica Training in Chennai
very nice blogs!!! i have to learning for lot of information for this sites...Sharing for wonderful information.Thanks for sharing this valuable information to our vision. You have posted a trust worthy blog keep sharing.
ReplyDeleteInformatica Training in Chennai
I am reading your post from the beginning, it was so interesting to read & I feel thanks to you for posting such a good blog, keep updates regularly.
ReplyDeleteinformatica training in chennai
Excellent article!!! LoadRunner is popular automation testing tool used for validating a software application/system under load. It delivers most precise information about the performance, functionality and behavior of the software product. Loadrunner Course in Chennai | Loadrunner training institute in Chennai
ReplyDeleteI am reading your post from the beginning, it was so interesting to read & I feel thanks to you for posting such a good blog, keep updates regularly.
ReplyDeleteRegards,
Angularjs training in chennai|Angularjs training chennai
http://www.webtrackker.com/erp_sap_Training_Course_institute_noida_delhi.php
ReplyDeletesap training institute in noida
ReplyDeleteAddress: php training institute in noida
ReplyDeleteWebtrackker Technologies
B-47, Sector- 64
Noida- 201301
Phone: 0120-4330760 , 8802820025
Email: Info@Webtrackker.Com
Web: www.webtrackker.com
Really awesome blog. Your blog is really useful for me. Thanks for sharing this informative blog. Keep update your blog.
ReplyDeleteOracle Training In Chennai
ReplyDeleteWeb designing Training Institute in noida - with 100% placement support - web trackker is the is best training institute for web designing, web development in delhi. In you are looking web designing Training in noida, web designing Training Institute in Noida, web designing training in delhi, web design training institute in Ghaziabad, web designing training institute, web designing training center in noida, web designing course contents, web designing industrial training institute in delhi, web designing training coaching institute, best training institute for web designing training, top ten training institute, web designing training courses and content then Webtrackker is the best option for you.
Linux Training Institute in Noida
ReplyDeleteBest Linux & UNIX Training Institute In Noida, Delhi- Web Trackker Is The Best Linux & Unix Training Institute In Noida, Top Linux & Unix Coaching In Noida Sector 63, 53, 18, 15, 16, 2, 64 Providing The Live Project Based Linux & Unix Industrial Training.
This comment has been removed by the author.
ReplyDeleteJava training institute in noida-webtrackker is best java training institute in noida witch also provides real time working trainer, then webtrackker best suggestion of you and better carrier if you are looking the"Java Training in Noida, java industrial training, java, j2ee training courses, java training institute in noida, java training center in delhi ncr, java training institute in ncr, Ghaziabad, project based java training, institute for advance java courses, training institute for advance java, java industrial training in noida, java/j2ee training courses in ghaziabad, meerut, noida sector 64, 65, 63, 15, 18, 2"Webtrackker is best otion for you.
ReplyDeleteJava training institute in noida-webtrackker is best java training institute in noida witch also provides real time working trainer, then webtrackker best suggestion of you and better carrier if you are looking the"Java Training in Noida, java industrial training, java, j2ee training courses, java training institute in noida, java training center in delhi ncr, java training institute in ncr, Ghaziabad, project based java training, institute for advance java courses, training institute for advance java, java industrial training in noida, java/j2ee training courses in ghaziabad, meerut, noida
ReplyDeletesector 64, 65, 63, 15, 18, 2"Webtrackker is best otion for you.
1800-640-8917 Norton antivirus technical support phone number, Norton customer support toll free number, NORTON antivirus customer Support number, 1800-640-8917 NORTON antivirus tech support number, Norton antivirus technical support phone number, 1800-640-8917 Norton antivirus technical support number, 1800-640-8917 Norton antivirus technical support toll free number, Norton technical support number.
ReplyDeleteWebtrackker Technologies- Webtrackker is an IT company and also provides the project based industrial training in Java, J2EE. Webtrackker also provide the 100% job placement support. JAVA Training Institute in Meerut, Best J2EE training Institute in Meerut, best JAVA Training Institute in Meerut, best JAVA Training Institute in Meerut, project JAVA Training in Meerut, JAVA Training on live project based in Meerut, Java Training Courses in Meerut, Summer Training Program in Meerut, Summer Training Program on java in Meerut.
ReplyDeleteWebtrackker Technologies- Webtrackker is an IT company and also provides the project based industrial training in Java, J2EE. Webtrackker also provide the 100% job placement support. JAVA Training Institute in Meerut, Best J2EE training Institute in Meerut, best JAVA Training Institute in Meerut, best JAVA Training Institute in Meerut, project JAVA Training in Meerut, JAVA Training on live project based in Meerut, Java Training Courses in Meerut, Summer Training Program in Meerut, Summer Training Program on java in Meerut.
ReplyDeletesas training institute in noida - web trackker is the best institute for industrial training for SAS in noida,if you are interested in SAS industrial training then join our specialized training programs now. webtrackker provides rel time working trainer with 100% placment suppot.
ReplyDeletesas training institute in noida - web trackker is the best institute for industrial training for SAS in noida,if you are interested in SAS industrial training then join our specialized training programs now. webtrackker provides real time working trainer with 100% placment suppot.
ReplyDeleteParis airport transfer - Parisairportransfer is very common in Paris that provides facilities to both the businessmen and the tourists. We provide airport transfers from London to any airport in London and also cruise transfer services at very affordable price to our valuable clients.
ReplyDeleteParis taxi
Paris airport shuttle
paris hotel transfer
paris airport transfer
paris shuttle
paris car service
paris airport service
disneyland paris transfer
paris airport transportation
beauvais airport transfer
taxi beauvais airport
taxi cdg airport
taxi orly airport
Wonderful concept. I like your post. Thanks for sharing.
ReplyDeletePrimavera Training in Kuwait
Thanks for the Tutorial..
ReplyDeleteDot Net Training in Chennai
Webtrackker Technology is a top leading IT company which is deal in all type of software and website development. Webtrackker also give the live project base Training on Linux. If you are looking the Linux Training Institute in Noida then Webtrackker is the best option for you.
ReplyDeleteLinux Training Institute in Noida
Java Training Institute in Noida - Croma Campus imparts the most effective JAVA Training in Noida which is based on the principle write once and run anywhere which means that the code which runs on one platform does not need to be complied again to run on the other.
ReplyDeleteJava Training Institute in Noida - Croma Campus imparts the most effective JAVA Training in Noida which is based on the principle write once and run anywhere which means that the code which runs on one platform does not need to be complied again to run on the other.
ReplyDeleteRobotics Training institute in Noida - Croma campus is a leading technical education institute in India provides robotics, Aero Models and Robotic training in noida from primary school to college students. We offer wide range of knowledge services and practicum on Robotics, complete understanding of the Robotic with our Class Room Training. Croma campus one of the best Robotic Training course provides a series of sessions & Lab Assignments which introduce and explain
ReplyDeleteGood job!
ReplyDeleteVery nice to read - with lots of explanations.
be projects in chennai
Informatica training institutes in noida - Croma campus offers best Informatica Training in noida with most experienced professionals. Our Instructors are working in Informatica and joint technologies for more years in MNC’s. We aware of industry needs and we are offering Informatica Training in noida.
ReplyDeleteInformatica training institutes in noida - Croma campus offers best Informatica Training in noida with most experienced professionals. Our Instructors are working in Informatica and joint technologies for more years in MNC’s. We aware of industry needs and we are offering Informatica Training in noida.
ReplyDeleteCroma campus has been NO.1 & Best Android training institute in noida offering 100% Guaranteed JOB Placements, Cost-Effective, Quality & Real time Training courses Croma campus provide all IT course like JAVA, DOT NET, ANDROID APPS, PHP, PLC SCADA, ROBOTICS and more IT training then joining us Croma campus and your best futures.
ReplyDeleteCroma campus best IT training course provide and best class Robotics trainer with job placement support. Robotics training in noida and best guidelines used by our programmers working on Robotics
ReplyDeleteCroma campus provides best class embedded system trainer with job placement support croma campus an IT training institute in noida best course in Embedded systems training in noida most of demanded course in IT fields.
ReplyDeleteExcellent post!!!.
ReplyDeletelenovo thinkpad service centers
lenovo ideapad service center
good.
ReplyDeleteoracle training in chennai
nice.
ReplyDeletesas training in chennai
Are you looking to make money from your visitors using popup ads?
ReplyDeleteIf so, have you ever used Ero-Advertising?
Croma campus noida best IT training institute and provide best class java trainer with 100% placement support.are you ready for java training join us croma campus java training institute in noida
ReplyDeleteCroma campus noida best IT training institute and provide best class java trainer with 100% placement support.are you ready for java training join us croma campus java training institute in noida
ReplyDeleteAndroid training in noida - Croma campus best training institute then Android is the quickest developing PDA OS on the planet today Android programming dialect is supported and created by Google and Open Handset Alliance and croma campus provide best android trainer with job placement support.
ReplyDeletePhp training institute in noida – Croma campus is best IT lending training institute php is most demanded IT sector join us croma campus training institute in noida
ReplyDeleteThis post is really nice and informative. The explanation given is really comprehensive and informative..
ReplyDeleteperl training in chennai
This is extremely helpful info!! Very good work. Everything is very interesting to learn and easy to understood. Thank you for giving information. Best Dot Net Training in Chennai
ReplyDeleteNice Informative blog - Best QTP Training In Chennai
ReplyDeleteVery Glad to find this post thanks for sharing this amazing articles -Sharepoint Training in Chennai
ReplyDeleteHighly informative post, visit: Best Labview Online Training
ReplyDeleteThank you for sharing your useful information.
ReplyDeleteload runner training in chennai
Thanks Admin for sharing ...
ReplyDeletesql-dba training in chennai
I have read your blog its very attractive and impressive. I like it your blog.
ReplyDeleteSpring online training Spring online training Spring Hibernate online training Spring Hibernate online training Java online training
spring training in chennai spring hibernate training in chennai
nice and great blog to read
ReplyDeletejava j2ee training course contents | java j2ee training institutes in chennai | java j2ee training | java training in chennai velachery
Thanks for sharing this information and keep updating us. This is more informatics and it really helped me to know the java developing.
ReplyDeleteJava Training in Chennai
Best JAVA Training in Chennai
Fastest Local Search engine in India
ReplyDeleteCALL360Leading Business Directory in India
This is an awesome post. Really very informative and creative contents. These concept is a good way to enhance the knowledge. Excellent post. Our Digital Marketing Training is tailored for beginners who want to learn how to stand out digitally, whether it is for their own business or a personal brand.
ReplyDeletedigital marketing training institute in chennai
digital marketing course fee in chennai
digital marketing course in velachery
is digital marketing a good career
best seo training center in chennai
digital marketing company in chennai
digital marketing agency in chennai
top digital marketing companies in chennai
Thanks for sharing this information its very useful info for us, Hi We at Colan Infotech Private Limited a
ReplyDeleteMobile application development company in chennai,
is Situated in US and India, will provide you best service in
enterprise mobile app development company .
We are one of the best software testing services company
ReplyDeleteand of course we stepped in bangalore too we are best qa and testing services
can provide quality assurance and testing services,
we are the best among the software testing company india
Thanks for sharing the useful information ,Hi We at Colan Infotech Private Limited a
ReplyDeleteMobile application development company in chennai,
is Situated in US and India, will provide you best service in
enterprise mobile app development company .
and Colan Infotech has a group of exceedingly dedicated, inventive and creative experts with an energy for delivering exciting , helpful and stylish Web and Mobile Applications, We work with customers in a wide variety of sectors.
We design all of our websites and applications using the responsive web design approach. Our talented team can handle all the aspects of mobility so we are rated as best service provider in
Mobile apps development companies in chennai.
We solidly trust that our customers start things out and there is not a viable alternative for quality of service.
We offer custom services to a wide range of industries by exceeding our client’s expectations. You can even interact directly with the team regarding your project, just as you would with your in-house team. we always desire to solicit our customer's fruitful experience with us, we are the top notch
Mobile App Development Company in chennai
and mobile app development companies in Bangalore. We can provide best
mobile app development chennai .
We can provide cutting edge technology services in
Mobile application development in chennai.
Reach us for mobile app development chennai or just call us for best
mobile app developers in chennai
Thanks for sharing this its very useful article, Hi We at Colan Infotech Private Limited a
ReplyDeleteMobile application development company in chennai,
is Situated in US and India, will provide you best service in
enterprise mobile app development company .
and Colan Infotech has a group of exceedingly dedicated, inventive and creative experts with an energy for delivering exciting , helpful and stylish Web and Mobile Applications, We work with customers in a wide variety of sectors.
We design all of our websites and applications using the responsive web design approach. Our talented team can handle all the aspects of mobility so we are rated as best service provider in
Mobile apps development companies in chennai.
We solidly trust that our customers start things out and there is not a viable alternative for quality of service.
We offer custom services to a wide range of industries by exceeding our client’s expectations. You can even interact directly with the team regarding your project, just as you would with your in-house team. we always desire to solicit our customer's fruitful experience with us, we are the top notch
Mobile App Development Company in chennai
and mobile app development companies in Bangalore. We can provide best
mobile app development chennai .
We can provide cutting edge technology services in
Mobile application development in chennai.
Reach us for mobile app development chennai or just call us for best
mobile app developers in chennai
Thanks for sharing this great information article,
ReplyDeleteasp .net web development company
Hi we at Colan Infotech Private Limited , a company which is Situated in US and India, will provide you best java web service and our talented
ReplyDeletejava application development. team will assure you best result and we are familiar with international markets, We work with customers in a wide variety of sectors. Our talented team can handle all the aspects of
Java web application development,we are the best among the
Java development company.
We have quite an extensive experience working with
java development services.
we are the only Java application development company which offer custom services to a wide range of industries by exceeding our client’s expectations. You can even interact directly with the team regarding your project, just as you would with your in-house team.Our pro team will provide you the best
java appliaction development services.
We are best among the
java development companies in Chennai,
please review our customer feedbacks so that you may find a clue about us. If you want one stop solution for java development outsourcing, Colan infotech is the only stop you need to step in. Colan Infotech is the unique
java web development company.were our team of unique
java application developer
were ranked top in
java enterprise application development.
Awesome post, Thanks for sharing very useful information article.
ReplyDeleteasp .net web development company.
asp .net development services.
Custom application development company.
ReplyDeleteThis is excellent information. It is amazing and wonderful to visit your site.Thanks for sharing this information,this is useful to me...
Android training in chennai
Ios training in chennai
ReplyDeleteThis is excellent information. It is amazing and wonderful to visit your site.Thanks for sharing this information,this is useful to me...
Android Training in Chennai
Ios Training in Chennai
ReplyDeleteThis is excellent information. It is amazing and wonderful to visit your site.Thanks for sharing this information,this is useful to me...
Android Training in Chennai
Ios Training in Chennai
Great post! This is for a good information, It's very helpful for this blog. And great valu for these information.This is good work you and you are doing well.
ReplyDeleteDot Net Training in Chennai
This is a great article, I have been always to read something with specific tips! I will have to work on the time for scheduling my learning.
ReplyDeleteDot Net Training in Chennai
In this post you can mentioned the all over information about the spring security. it is very relevant for the J2EE-based enterprise software applications. in spring security it is mainly use for providing comprehensive security. it is the best part for security purpose. and thanks for sharing this post.
ReplyDeleteseo training in nagpur
Thanks for your informative articel .its very useful
ReplyDeletedot net training center in chennai | dot net training institute in velachery | dot net training and placement in chennai
Thanks for your informative articel .its very useful
ReplyDeletedot net training center in chennai | dot net training institute in velachery | dot net training and placement in chennai
Nice Post
ReplyDeleteEnterprise Software Applications
Useful article.. I learnt about spring mvc from this article and explanation also very clear so easy to understand.. thank you for sharing
ReplyDeletebest hadoop training in chennai | best big data training in chennai
Excellent tutorial work thanks for sharing...
ReplyDeleteBest Java Training in Chennai
You have explained the concept the responsive web design in this article, really informative keep sharing...
ReplyDeleteweb designing course in chennai|web designing training
Great effort you have taken. You have shared really useful program with us. Thanks to shared your knowledge with us.
ReplyDeleteOracle dba training | Oracle courses
Excellent article. Very interesting to read. I really love to read such a nice article. Thanks! keep rocking.I am learn lot from you in MVC tutorials...
ReplyDeleteQlikView Training in Chennai
Informatica Training in Chennai
Good Post
ReplyDeleteVmware Training in Chennai
CCNA Training in Chennai
Angularjs Training in Chennai
Google CLoud Training in Chennai
Red Hat Training in Chennai
Linux Training in Chennai
Rhce Training in Chennai
Pretty good post. I just stumbled upon your blog and wanted to say that I have really enjoyed reading your blog posts. Any way I'll be subscribing to your feed and I hope you post again soon. Big thanks for the useful info.
ReplyDeleteAngularJS Training in Chennai
thank you java training in chennai
ReplyDeletestruts training in chennai
core java training in chennai
ReplyDeleteThank you very muchjava projects in chennai final projects in chennai
cse projects in chennai me projects in chennai
btech projects in chennai mtech projects in chennai
Excellent article. Very interesting to read. I really love to read such a nice article. Thanks! keep rocking.
ReplyDeleteInformatica Training in Chennai
Python Training in Chennai
Best AngularJS Training in Chennai
Cool stuff you have got and you keep update all of us.
ReplyDeleteHadoop Training in Chennai
Big Data Training in Chennai
Python Training in Chennai
Python Training Centers in Chennai
Data Science Training in Chennai
Data Science Course in Chennai
Data Analytics Training in Chennai
Best AngularJS Training in Chennai
AngularJS Training in Chennai
QlikView Training in Chennai
Informatica Training in Chennai
Great post…. you have done great job…its very cool blog. Linking is very useful thing you have really helped lots of people who visit this blog and provided them this useful information on opinionated astrologer. Thanks a lot for this. Any way I’ll be subscribing to your feed and I hope you post again soon.
ReplyDeleteHadoop Training in Chennai
Big Data Training in Chennai
Python Training in Chennai
Python Training Centers in Chennai
Data Science Training in Chennai
Data Science Course in Chennai
Data Analytics Training in Chennai
Best AngularJS Training in Chennai
AngularJS Training in Chennai
QlikView Training in Chennai
Informatica Training in Chennai
Thank you so much for sharing... Lucky Patcher windows
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteNice Post....Good For Reading
ReplyDeleteSalesforce Training Online
Refer this for more updates
ReplyDeleteRisk management consulting services
ROI consultant minnesota
consulting company minnesota
AC Mechanic in Chennai
ReplyDeleteAuditoriums in Chennai
Automobile Batteries in Chennai
Automobile Spares in Chennai
Money Exchange in Chennai
Soft Skills Academy Chennai
Ceramics Showroom in Chennai
Yoga Class Chennai
Ladies Hostel Chennai
Computer Sales and Service
Excellent Article
ReplyDeleteAC Mechanic in Anna Nagar
Excellent artical
ReplyDeleteSalesforce developer training
Salesforce
Thnaks
Hi Krams,
ReplyDeleteThis is one awesome blog. Much thanks again. Fantastic.
We have a requirement like, we send the DocuSign request from and we check whether the request is successful or not from both Desktop and Mobile versions. In Desktop version, we are printing the page level err message after getting the failure response from DocuSign but the same thing we want to achieve it in mobile side as well. I know we can't print the page level messages in mobile but we can give some alert to the user on the failure side. Can someone please help me on how to get the call back response in this mobile context?
But great job man, do keep posted with the new updates.
Many Thanks,
Clinton Kevin
Hi
ReplyDeleteThanks for the information it was very helpful. Here i am sharing the link of important Java Spring interview questions
Your new valuable key points imply much a person like me and extremely more to my office workers. With thanks; from every one of us.
ReplyDeleteBest Java Training Institute in chennai
Finding the time and actual effort to create a superb article like this is great thing. I’ll learn many new stuff right here! Good luck for the next post buddy..
ReplyDeleteBest Industrial Training in Noida
Best Industrial Training in Noida
Nice Post. Thank you for sharing with us.
ReplyDeleteAndroid Training in Chennai
Best Android Training in Chennai
Really it was an awesome article...very interesting to read..You have provided an nice article....Thanks for sharing.
ReplyDeleteBest BCA Colleges in Noida
I have read your blog its very attractive and impressive. I like it your blog.
ReplyDeleteBest BCA Colleges in Noida
Nice article Thanks for sharing this article Wonderful information from this website Thanks !!!
ReplyDeleteEmbedded system training in chennai | PLC training institute in chennai
Greetings from Florida! I’m bored at work, so I decided to browse your site on my iPhone during lunch break. I love the information you provide here and can’t wait to take a look when I get home. I’m surprised at how fast your blog loaded on my cell phone .. I’m not even using WIFI, just 3G. Anyways, awesome blog!
ReplyDeleteDevOps Training in Chennai
Thanks for Sharing with us.
ReplyDeleteSoftware Training in Chennai
Thank you Admin!
ReplyDelete1 crore projects center specializes in Embedded software Training in chennai